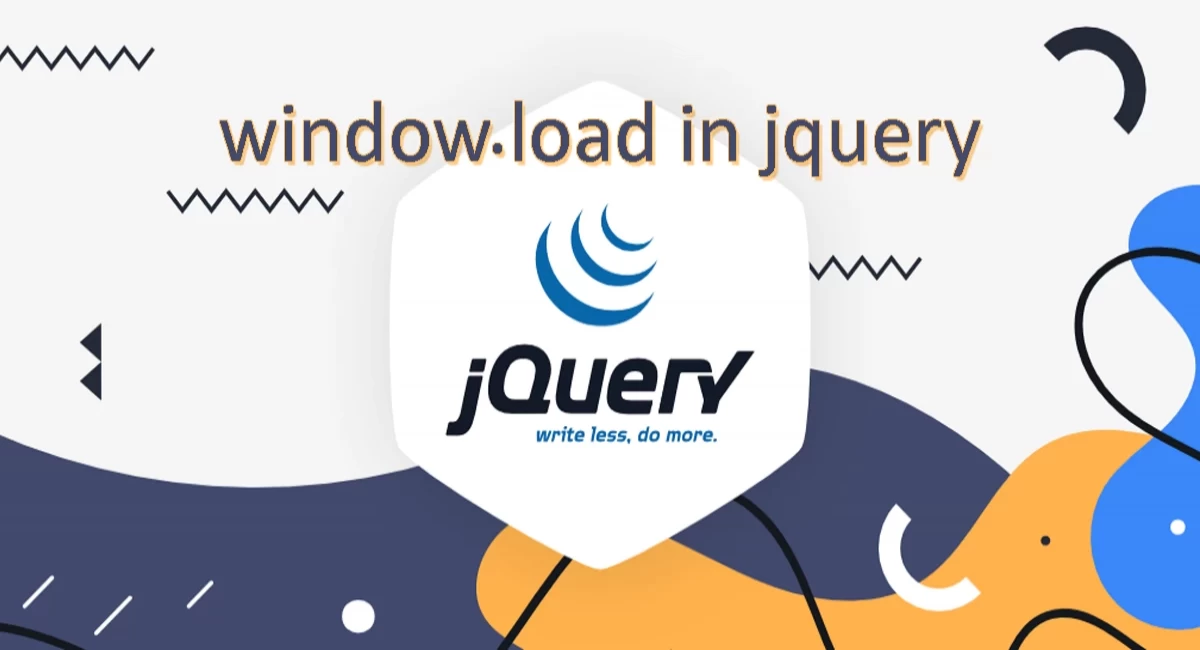
Guide to $(window).load() Method in jQuery
Hello there! 👋 Welcome to our informative blog post about the $(window).load() method in jQuery! If you're new to web development or just starting with jQuery, you might have come across this term and wondered what it means. Don't worry, we've got you covered! In this article, we will explain what the $(window).load() method is all about and how it can benefit your web development projects. Let's dive in! 😊
What is the $(window).load() Method in jQuery?
The $(window).load() method in jQuery is an event that is triggered when all the content of a web page, including images, stylesheets, and scripts, has finished loading. It provides a convenient way to execute code or perform actions only after the entire web page has fully loaded. By using this method, you can ensure that all the elements on the page are available for manipulation, resulting in a smoother and more interactive user experience.
Key Differences between $(window).load() and $(document).ready()
Before we explore the benefits of using $(window).load(), let's quickly discuss the differences between $(window).load() and $(document).ready() functions in jQuery. While both events are commonly used in web development, they serve different purposes.
The $(window).load() event is triggered after the entire web page, including external resources like images, has finished loading. On the other hand, the $(document).ready() event is fired when the HTML document has been completely parsed and loaded, without waiting for external resources.
In simple terms, $(window).load() waits for everything to load, including images, while $(document).ready() fires as soon as the HTML structure is ready. If your code needs access to external resources like images, you should use $(window).load(), whereas if you only require access to the DOM structure, $(document).ready() is sufficient.
Example 1: Displaying an Alert on Page Load
Let's explore a practical example to demonstrate the usage of $(window).load(). Suppose you want to display a friendly greeting to your users using an alert box once your web page has finished loading. Here's how you can achieve this using jQuery:
$(window).on('load', function() {
alert('Welcome to our website! 🎉');
});
In this example, we're using the $(window).on('load', ...) syntax, which binds an event handler function to the window's load event. When the load event is triggered, the function inside the callback will be executed, displaying a welcome message in an alert box. This ensures that the alert will only be shown when all the content on the page, including images and other assets, has finished loading.
Example 2: Adding a Fade-in Effect to an Image
Let's consider another example where you want to add a fade-in effect to an image once it's fully loaded. You can utilize $(window).load() to achieve this effect. Here's an example:
<img id="myImage" src="image.jpg" style="display:none;">
$(window).on('load', function() {
$('#myImage').fadeIn(1000); // Fade in the image over 1 second
});
In this example, we have an image element with an id of "myImage" and an inline style of "display:none;" to initially hide it. When the window's load event is triggered, the image will start to fade in gradually over 1 second (1000 milliseconds) using the fadeIn() method provided by jQuery. This ensures that the fade-in effect will only be applied once the image has finished loading.
How to Fix the "window.load jQuery not Working" Issue
If you're experiencing issues with the window.load jQuery function not working as expected, there are a few steps you can take to resolve it. Here are some tips:
- Check the order of your script tags: Ensure that the script tag for the jQuery library is placed before the script tag for your custom jQuery code. This ensures that the jQuery library is loaded and available before your code tries to use it.
- Use the shorthand syntax: Instead of using the $(window).load() method, consider using the shorthand syntax $(window).on("load", function() {}). This syntax is less likely to encounter conflicts with other JavaScript libraries and can help resolve compatibility issues.
- Check for errors: Use your browser's developer tools to check for any JavaScript errors in the console. Fixing these errors can often resolve issues with jQuery functions not working properly.
- Update your jQuery version: If you're using an older version of jQuery, consider updating it to the latest stable release. Newer versions may include bug fixes and improvements that can address compatibility issues.
By following these tips, you should be able to troubleshoot and fix the "window.load jQuery not working" issue and ensure that your jQuery code functions properly.
Conclusion
We hope this comprehensive guide to the $(window).load() method in jQuery has provided you with a clear understanding of its purpose and benefits. By utilizing this event, you can execute code or perform actions only after the entire web page, including images and external resources, has finished loading.
Remember to use $(window).load() responsibly and avoid unnecessary usage, as it can impact the performance of your web page. Additionally, keep in mind that the load event may take longer to trigger on slower connections or heavier web pages due to the loading time of external assets.
If you have any further questions or need clarification, feel free to leave a comment below. Happy coding! 🚀
Comments
No comments yet.
Add Comment