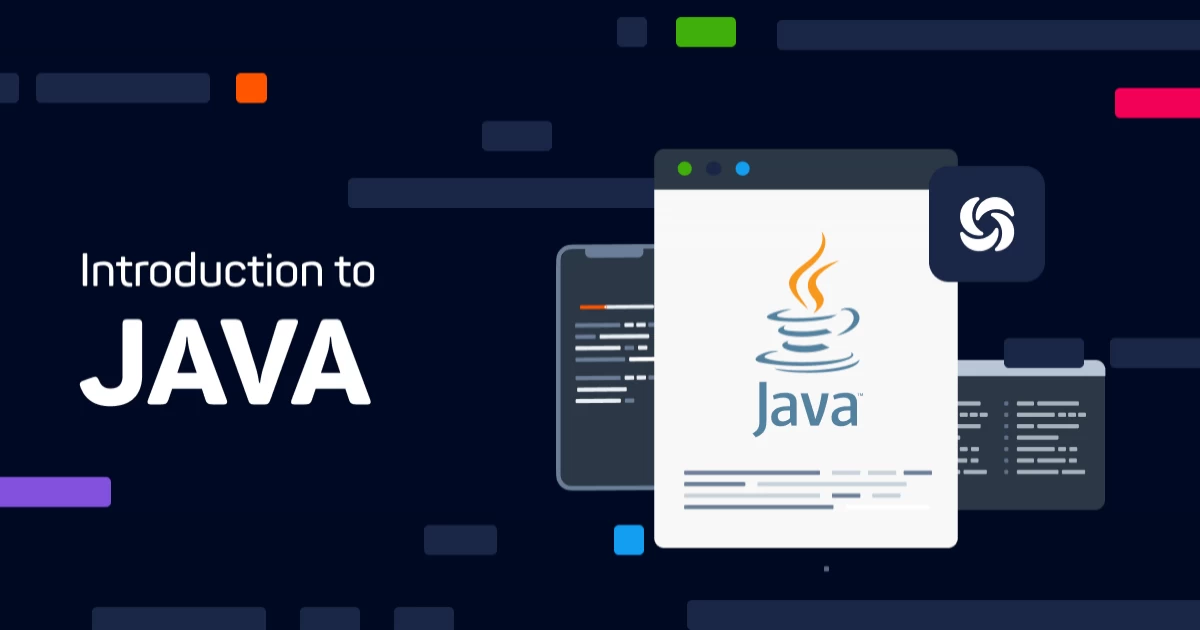
An Introduction to Java Programming Language | Learn About Java Basics
Java is a popular programming language that is widely used in the development of web applications, mobile apps, and desktop software. It was created by James Gosling at Sun Microsystems (now part of Oracle) in 1995 and has since become one of the most widely used programming languages in the world.
Basic Syntax
Java syntax is similar to C++, but it has some differences that make it more accessible to beginners. Here's an example of a "Hello, World!" program in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
The above code defines a class called "HelloWorld" and a method called "main" which prints the string "Hello, World!" to the console. The "public" keyword is an access modifier that allows the class to be accessed from outside the package.
Data Types
Java supports several data types, including integers, floating-point numbers, booleans, and characters. Here's an example of how to declare a variable and assign it a value:
int myNumber = 42;
float myFloat = 3.14f;
boolean myBoolean = true;
char myChar = 'a';
The above code declares four variables of different data types and assigns them values. Note that the "f" suffix is used to indicate that the floating-point number is a "float" rather than a "double".
Control Flow Statements
Java supports several control flow statements, including "if" statements, "for" loops, and "while" loops. Here's an example of an "if" statement:
int x = 10;
if (x > 5) {
System.out.println("x is greater than 5");
}
The above code checks if the variable "x" is greater than 5 and prints a message to the console if it is. Here's an example of a "for" loop:
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
The above code iterates over the values 0 through 4 and prints each value to the console.
Conclusion
Java is a powerful and versatile programming language that is used by developers all over the world. It has a straightforward syntax, supports many data types and control flow statements, and can be used to develop a wide range of applications. If you're interested in learning more about Java, there are many resources available online to help you get started.
Comments
on 2023-03-24 11:36:35
on 2023-03-24 11:40:12
on 2023-03-24 11:41:39
on 2023-03-24 18:49:05
Add Comment