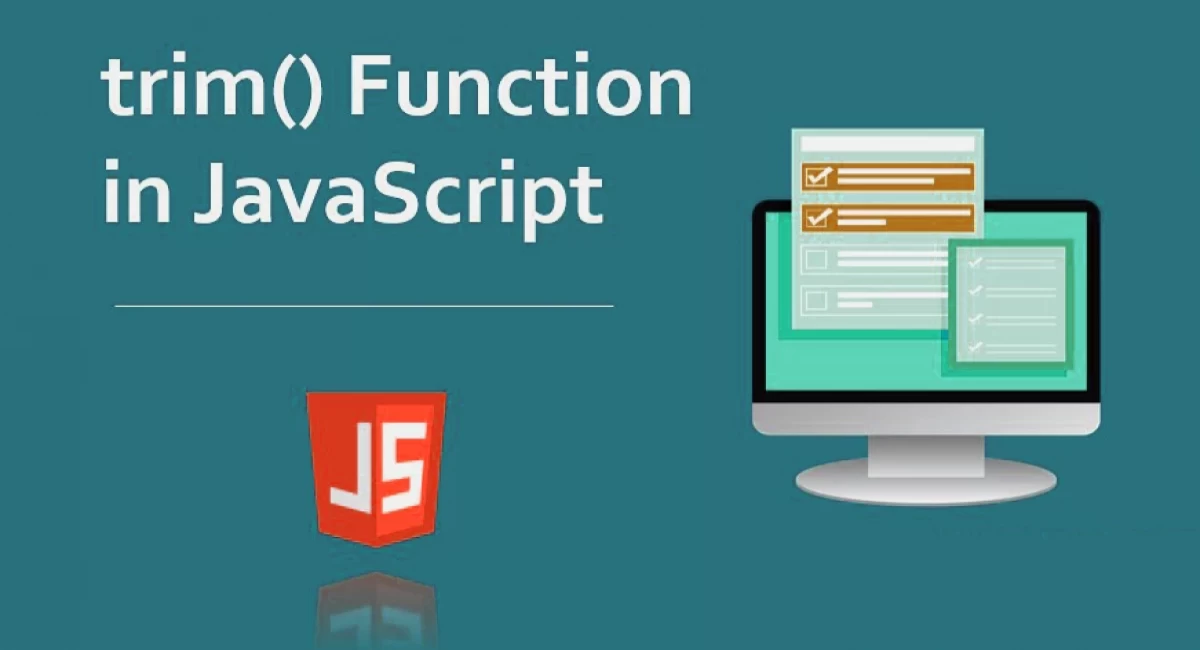
Trim Function in jQuery and JavaScript for Old Browsers - Tips and Tricks
The "trim" function is used to remove white spaces (spaces, tabs, and newlines) from both sides of a string in jQuery and JavaScript. This is particularly useful when you're dealing with user inputs or extracting data from APIs that may contain unwanted spaces.
In jQuery, you can use the "trim" function as follows:
var str = " Hello, World! ";
var trimmed = $.trim(str);
console.log(trimmed); // Output: "Hello, World!"
As you can see, we're using the "$.trim" function here, which is a shorthand for "jQuery.trim". This function removes the white spaces from both sides of the "str" variable and returns the trimmed string.
In JavaScript, you can use the "trim" function as follows:
var str = " Hello, World! ";
var trimmed = str.trim();
console.log(trimmed); // Output: "Hello, World!"
Here, we're calling the "trim" function directly on the "str" variable. This function is available in modern browsers, but older browsers may not support it.
So, how do we use the "trim" function for old browsers? One way to do this is by creating a custom function that mimics the behavior of the "trim" function. Here's an example:
function trim(str) {
return str.replace(/^\s+|\s+$/g, '');
}
var str = " Hello, World! ";
var trimmed = trim(str);
console.log(trimmed); // Output: "Hello, World!"
Here, we're creating a custom "trim" function that uses a regular expression to remove white spaces from both sides of the string. The regular expression "/^\s+|\s+$/g" matches one or more white spaces at the beginning or end of the string and replaces them with an empty string.
In conclusion, the "trim" function is a useful tool for removing unwanted white spaces from strings. In jQuery, you can use the "$.trim" function, and in JavaScript, you can use the "trim" function. If you're dealing with old browsers that don't support the "trim" function, you can create a custom function that mimics its behavior using regular expressions. Happy coding!
Comments
No comments yet.
Add Comment