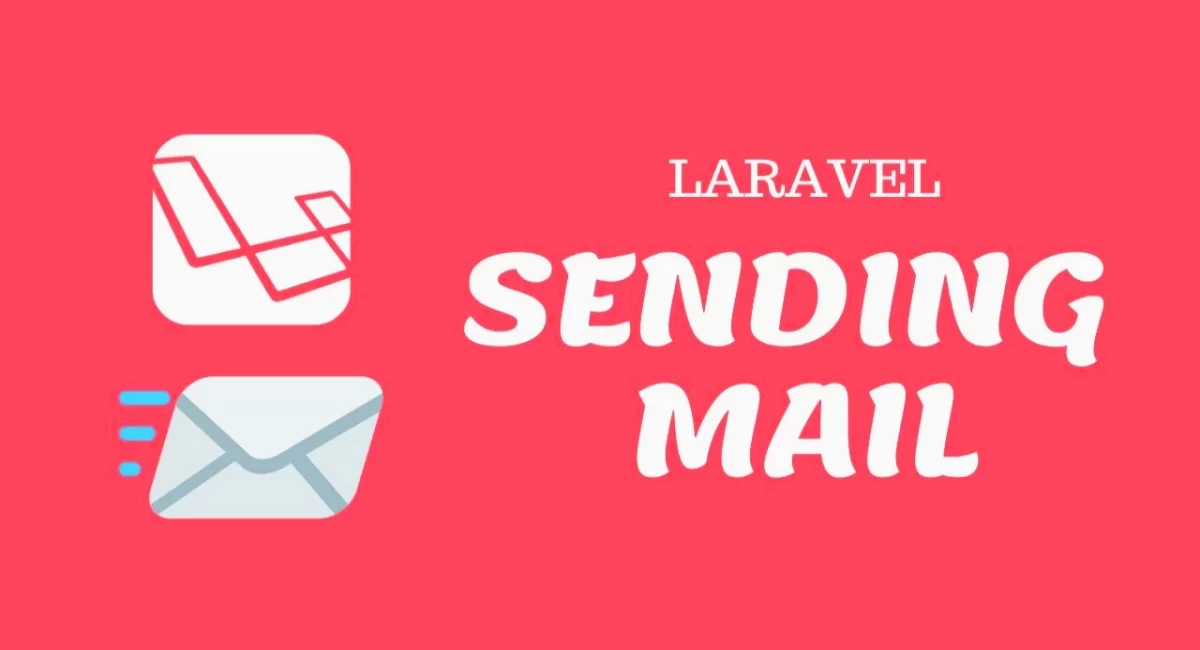
How to Send Mail in Laravel
Sending emails is an essential part of modern web development. Laravel, a PHP framework, provides built-in support for sending emails via a variety of drivers, including SMTP, Mailgun, Mandrill, and Amazon SES. In this article, we'll show you how to send mail in Laravel and cover some basic and advanced features that you can use to customize and enhance your email functionality.
Step 1: Install Laravel
If you haven't already done so, the first step is to install Laravel. You can do this using composer by running the following command:
composer create-project --prefer-dist laravel/laravel your-project-name
This command will create a new Laravel project in a directory called your-project-name.
Step 2: Configure Your Mail Settings
Before you can send email, you need to configure your mail settings. Laravel provides a simple way to do this using environment variables. Open the .env file in your project root directory and update the following variables:
MAIL_DRIVER=smtp
MAIL_HOST=smtp.gmail.com
MAIL_PORT=587
MAIL_USERNAME=your-email@gmail.com
MAIL_PASSWORD=your-gmail-password
MAIL_ENCRYPTION=tls
These settings will configure Laravel to use the Gmail SMTP server to send email. Make sure to replace the MAIL_USERNAME and MAIL_PASSWORD values with your actual Gmail account details.
Step 3: Send Your First Email
Now that your mail settings are configured, you can start sending email. Laravel provides a simple way to send email using the Mail facade. Here's an example:
use Illuminate\Support\Facades\Mail;
use App\Mail\WelcomeEmail;
Mail::to('user@example.com')->send(new WelcomeEmail());
In this example, we're using the Mail facade to send an email to user@example.com. We're also passing a WelcomeEmail instance to the send() method. Here's what the WelcomeEmail class might look like:
use Illuminate\Bus\Queueable;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
use Illuminate\Contracts\Queue\ShouldQueue;
class WelcomeEmail extends Mailable
{
use Queueable, SerializesModels;
public function build()
{
return $this->view('emails.welcome');
}
}
This class extends the Mailable class provided by Laravel and implements the ShouldQueue interface, which allows the email to be sent asynchronously. In the build() method, we're returning a view called emails.welcome, which is where the email content will be defined.
Step 4: Customize Your Email
Laravel provides a variety of methods for customizing your email. Here are a few examples:
Adding Attachments
You can add attachments to your email using the attach() method. Here's an example:
public function build()
{
return $this->view('emails.welcome')
->attach('/path/to/file');
}
This example attaches a file located at /path/to/file to the email.
Sending Markdown Emails
You can use Laravel's built-in Markdown support to send beautifully formatted emails. Here's an example:
public function build()
{
return $this->markdown('emails.welcome');
}
This example uses the markdown() method to specify that the email content should be rendered using a Markdown view. You can create your Markdown views in the resources/views directory using the .md file extension.
Using Custom SMTP Servers
If you don't want to use the built-in mail drivers provided by Laravel, you can use the SMTP driver to send email via a custom SMTP server. Here's an example:
MAIL_DRIVER=smtp
MAIL_HOST=smtp.example.com
MAIL_PORT=587
MAIL_USERNAME=your-username
MAIL_PASSWORD=your-password
MAIL_ENCRYPTION=tls
In this example, we're configuring Laravel to use an SMTP server located at smtp.example.com. You'll need to replace the MAIL_USERNAME and MAIL_PASSWORD values with your actual SMTP server credentials.
Step 5: Conclusion
In this article, we've shown you how to send mail in Laravel using the built-in Mail facade. We've also covered some basic and advanced features, such as file uploads, custom SMTP servers, and Markdown support. With these tools, you can easily create customized email functionality for your Laravel applications.
If you want to learn more about Laravel's email functionality, check out the official Laravel documentation at https://laravel.com/docs/mail.
Comments
No comments yet.
Add Comment