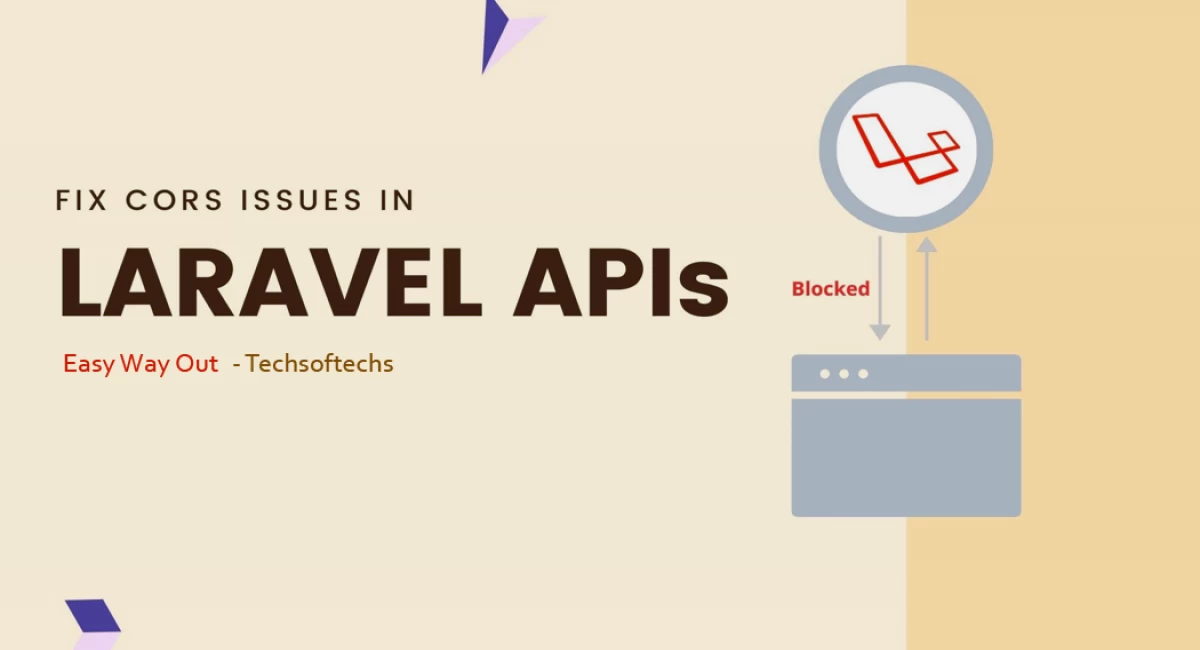
Resolving CORS Issue in Laravel API Development
Hey Folks!π
Are you encountering CORS (Cross-Origin Resource Sharing) issues while developing your API in Laravel? Don't worry; you're not alone! CORS problems can be frustrating, but I've got you covered with a step-by-step guide on how to resolve them seamlessly.
Understanding the CORS Issue
CORS issues often arise when your frontend application, hosted on one domain, tries to access resources (APIs) located on another domain. This security feature implemented by browsers can sometimes lead to blocked requests and errors.
Step 1: Implementing CORS Middleware
To tackle CORS issues effectively, let's create a custom middleware named Cors.php. This middleware will handle CORS headers for incoming requests.
<?php
namespace App\Http\Middleware;
use Closure;
class Cors
{
public function handle($request, Closure $next)
{
return $next($request)
->header('Access-Control-Allow-Origin', '*')
->header('Access-Control-Allow-Methods', '*')
->header('Access-Control-Allow-Headers', '*');
}
}
Step 2: Integrating Middleware into Kernel.php
Once the middleware is defined, integrate it into your application's HTTP kernel.
<?php
class Kernel extends HttpKernel
{
protected $middleware = [
\App\Http\Middleware\Cors::class,
// Add other middleware as needed
];
}
Step 3: Creating a Response Function
For a more streamlined approach, let's create a response function that automatically includes CORS headers.
<?php
class YourAPIController extends Controller
{
private function createResponse($data)
{
$response = response()->json($data);
$response->header('Access-Control-Allow-Origin', '*');
$response->header('Access-Control-Allow-Methods', 'GET, POST, PUT, DELETE, OPTIONS');
$response->header('Access-Control-Allow-Headers', 'Content-Type, Authorization');
return $response;
}
public function my_api()
{
return $this->createResponse(['status' => 1, 'message' => 'API Success']);
}
}
If You Lost in the labyrinth of Laravel middleware? No worries, mate! Click here π to discover where to stash that sneaky Cors.php
and tame it into your Kernel. It's like uncovering buried treasure, but with fewer pirates and more code! π»β
Bonus Tip: Authorization Verification
Enhance your API's security by verifying authorization tokens in the controller constructor.
<?php
class YourAPIController extends Controller
{
public function __construct()
{
$this->middleware(function ($request, $next) {
$token = $request->bearerToken();
if (!$token) {
return response()->json(['message' => 'Unauthenticated.'], 401);
}
$user = DB::table('users')->where('token', $token)->first();
if (!$user) {
return response()->json(['message' => 'Invalid token.'], 401);
}
return $next($request);
});
}
}
Note: To prevent CORS conflicts, consolidate CORS configuration into one place, preferably in Laravel middleware, and remove CORS-related directives from the `.htaccess` file.
Conclusion:
By following these steps, you can effectively resolve CORS issues in your Laravel API development. Implementing CORS middleware, creating a response function, and adding bonus security measures will ensure smooth and secure communication between your frontend and backend. Happy Coding! π
Comments
No comments yet.
Add Comment