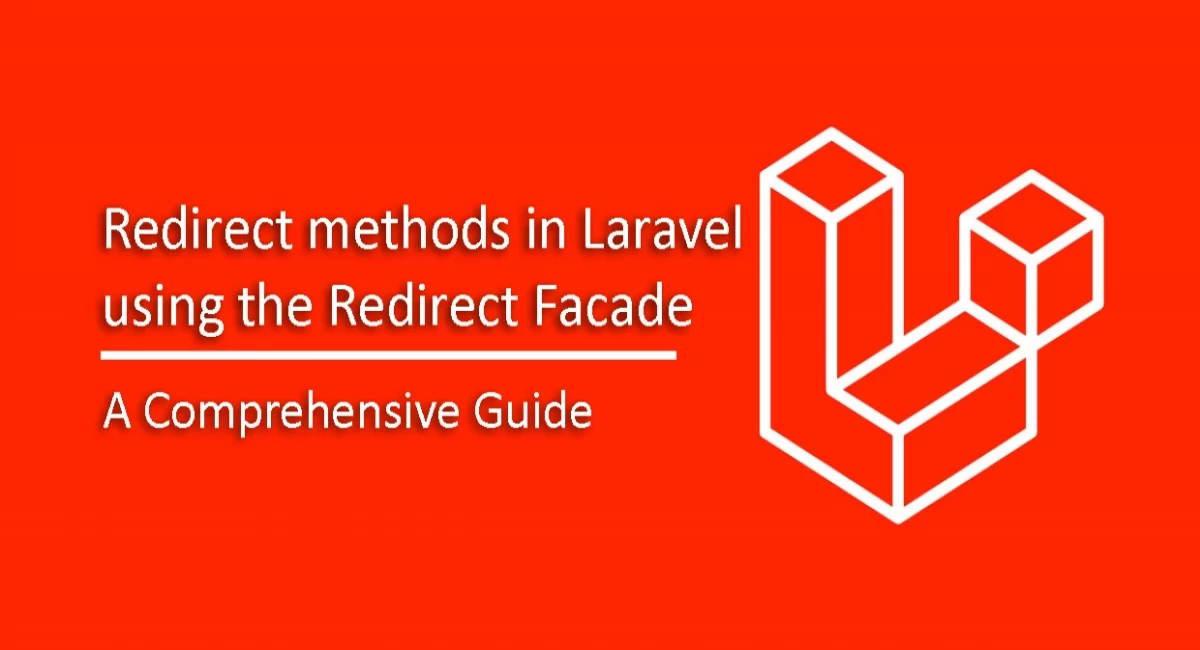
Laravel Redirect Methods Using the Redirect Facade
👋 Hey again! We're back with some additional information on redirects in Laravel.
🔀 In addition to the redirect() function and Redirect class, Laravel also provides the Illuminate\Support\Facades\Redirect facade to create redirect responses. Laravel's Redirect Facade is a powerful tool for redirecting users to different pages within your application. It provides several methods that make it easy to perform redirects in a variety of situations.
Redirecting with Redirect::to()
The most basic method provided by the Redirect Facade is the to()
function. This function accepts a single argument, which is the URL to which you want to redirect the user. Here's an example:
return Redirect::to('/dashboard');
In this example, we're redirecting the user to the /dashboard
URL. Laravel will automatically generate a redirect response with a 302 status code and the appropriate Location
header to send the user to the new URL.
Shortcut Redirects
In addition to the to()
function, Laravel also provides several shortcut methods for common redirects. For example, if you want to redirect the user back to the previous page they were on, you can use the back()
function:
return Redirect::back();
This will generate a redirect response with a 302 status code and the Referer
header set to the URL of the previous page.
Laravel also provides a route()
function for redirecting the user to a named route in your application. Here's an example:
return Redirect::route('dashboard');
In this example, we're redirecting the user to the dashboard
route, which we've defined elsewhere in our application using the Route::get()
function.
Redirecting with Data
In some cases, you may want to redirect the user to a new page and pass data along with the redirect. Laravel makes this easy using the with()
function. Here's an example:
return Redirect::to('/dashboard')->with('success', 'Your account has been updated.');
In this example, we're redirecting the user to the /dashboard
URL and passing along a success message with the key success
and the value Your account has been updated.
. We can then retrieve this message on the new page using the session()
function:
<div class="alert alert-success">
{{ session('success') }}
</div>
This will display the success message to the user on the new page.
Conclusion
The Redirect Facade is a powerful tool for redirecting users in Laravel. Whether you need to redirect the user to a new page, redirect them back to the previous page, or pass data along with the redirect, Laravel's Redirect Facade has you covered. By using the various functions provided by the Redirect Facade, you can easily perform common redirects and pass data between pages in your application.
Overall, the Redirect Facade is just one of the many features that make Laravel a popular and powerful PHP framework. If you're not already using Laravel, consider giving it a try and exploring the many other features it has to offer. Happy coding!
Comments
No comments yet.
Add Comment