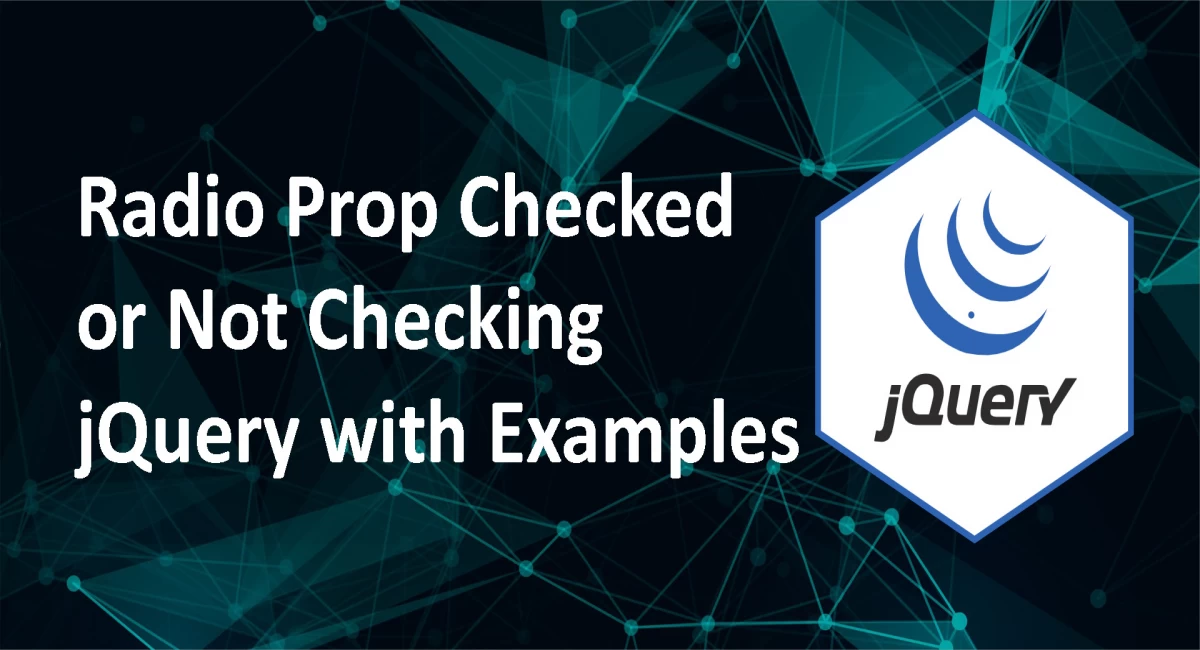
Radio Prop Checked or Not Checking jQuery with Examples
When working with forms on a webpage, you may need to check whether a radio button is checked or not. In jQuery, you can use the .prop()
method to get or set the value of the checked
property of a radio button.
Checking if a Radio Button is Checked or Not
To check if a radio button is checked or not using jQuery, you can use the .prop()
method to get the value of the checked
property. Here's an example:
<input type="radio" name="myRadio" value="1" id="myRadio1">
<input type="radio" name="myRadio" value="2" id="myRadio2">
// Select the radio button elements using their name attribute
var radioButtons = $('input[name="myRadio"]');
// Loop through each radio button element
radioButtons.each(function() {
var radioButton = $(this);
// Check if the radio button is checked
if (radioButton.prop('checked')) {
console.log('The radio button with value ' + radioButton.val() + ' is checked');
} else {
console.log('The radio button with value ' + radioButton.val() + ' is not checked');
}
});
This code selects the radio button elements using their name
attribute and loops through each element using the .each()
method. For each radio button element, the code checks the value of the checked
property using the .prop()
method. If the radio button is checked, the code logs a message to the console saying that the radio button is checked and displays its value. If the radio button is not checked, the code logs a message saying that the radio button is not checked and displays its value.
Setting the Checked Property of a Radio Button
To set the checked property of a radio button using jQuery, you can use the .prop()
method to set the checked
property to true
. Here's an example:
<input type="radio" name="myRadio" value="1" id="myRadio1">
<input type="radio" name="myRadio" value="2" id="myRadio2">
// Select the radio button element using its ID
var radioButton = $('#myRadio1');
// Set the radio button to checked
radioButton.prop('checked', true);
This code selects the radio button element using its ID and sets the checked
property to true
, which checks the radio button.
Conclusion
Using the .prop()
method in jQuery makes it easy to check whether a radio button is checked or not, and to set the checked property of a radio button. By using these methods, you can easily manipulate radio buttons on a webpage to create a better user experience for your users.
Comments
No comments yet.
Add Comment