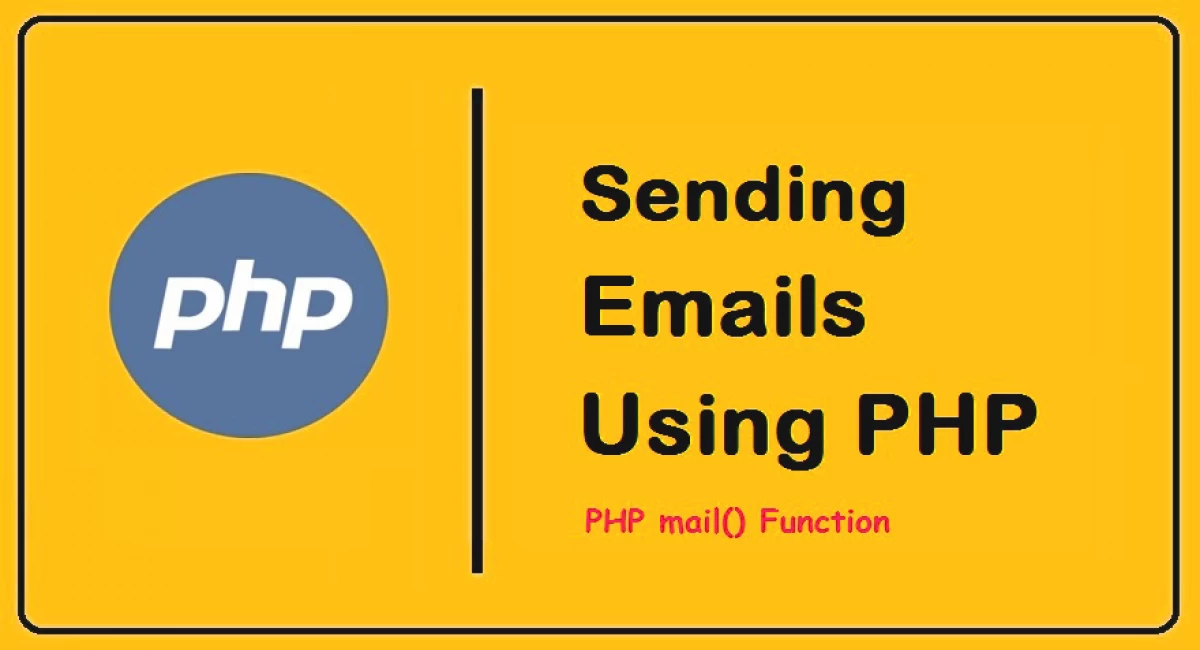
How to Send Mail in PHP with Content Type HTML | Tutorial
Sending emails is a common functionality in web development, and PHP provides a simple and efficient way to send emails using the mail()
function. In this blog post, we will explore how to send an email in PHP with the content type set to HTML.
Prerequisites
Before we begin, make sure you have a PHP-enabled server or local development environment set up. You can check if PHP is installed on your system by running the following command in the command line:
php -v
If PHP is not installed, you can download and install it from the official PHP website.
Sending HTML Emails
To send an email with HTML content, you need to set the appropriate headers and content type in the email headers. Here's an example:
<?php
$to = '[email protected]';
$subject = 'Example HTML Email';
$message = '<html><body><h1>Hello, World!</h1></body></html>';
$headers = 'MIME-Version: 1.0' . "\r\n";
$headers .= 'Content-type: text/html; charset=iso-8859-1' . "\r\n";
$headers .= 'From: [email protected]' . "\r\n";
if (mail($to, $subject, $message, $headers)) {
echo 'Email sent successfully!';
} else {
echo 'Email delivery failed.';
}
?>
In the code snippet above, we first set the recipient's email address, the subject of the email, and the HTML content of the message. Then, we define the email headers, including the MIME version, content type, and the "From" address.
We use the mail()
function to send the email. It takes the recipient, subject, message, and headers as parameters. If the email is sent successfully, the code will output "Email sent successfully!"; otherwise, it will display "Email delivery failed."
Additional Examples
Let's explore some additional examples that demonstrate various scenarios of sending emails in PHP.
Send an Email with Attachments
If you need to send an email with attachments, you can use the PHPMailer
library or a similar third-party library. Here's an example using PHPMailer
:
<?php
require 'PHPMailer/PHPMailer.php';
require 'PHPMailer/SMTP.php';
$mail = new PHPMailer\PHPMailer\PHPMailer();
$mail->isSMTP();
$mail->Host = 'smtp.example.com';
$mail->SMTPAuth = true;
$mail->Username = '[email protected]';
$mail->Password = 'your_email_password';
$mail->SMTPSecure = 'tls';
$mail->Port = 587;
$mail->setFrom('[email protected]', 'Sender Name');
$mail->addAddress('[email protected]', 'Recipient Name');
$mail->Subject = 'Example Email with Attachment';
$mail->Body = '<h1>Hello, World!</h1>';
$mail->isHTML(true);
$mail->addAttachment('/path/to/attachment.pdf');
if ($mail->send()) {
echo 'Email sent successfully!';
} else {
echo 'Email delivery failed.';
}
?>
In this example, we use the popular PHPMailer
library to send an email with an attachment. First, we include the necessary files from the library. Then, we create a new instance of the PHPMailer
class.
We set the SMTP server details, including the hostname, authentication credentials, and encryption method. Next, we specify the sender and recipient information, subject, and HTML body of the email. We enable HTML content with the isHTML(true)
method.
Finally, we add the attachment using the addAttachment()
method, providing the file path. We call the send()
method to send the email, and if successful, it will output "Email sent successfully!" Otherwise, it will display "Email delivery failed."
Send Email with Dynamic Content
You can personalize your emails by including dynamic content from variables or retrieving data from a database. Here's an example:
<?php
$to = '[email protected]';
$subject = 'Personalized Email';
$name = 'John Doe';
$message = "Hello, $name! This is an example of a personalized email.";
// Rest of the code remains the same
?>
In this example, we include a variable $name
and use it in the email message to personalize the greeting. You can retrieve dynamic data from user input, a database, or any other source to customize the email content.
Conclusion
Sending emails in PHP with HTML content type is a straightforward process. By setting the appropriate headers and using the mail()
function, you can send beautifully formatted HTML emails to recipients. Additionally, when advanced features like attachments are required, third-party libraries like PHPMailer
provide comprehensive solutions.
Remember to handle errors gracefully and ensure that your server is properly configured to send emails. With the knowledge gained from this blog post, you can now enhance your PHP applications by incorporating email functionality.
Comments
No comments yet.
Add Comment