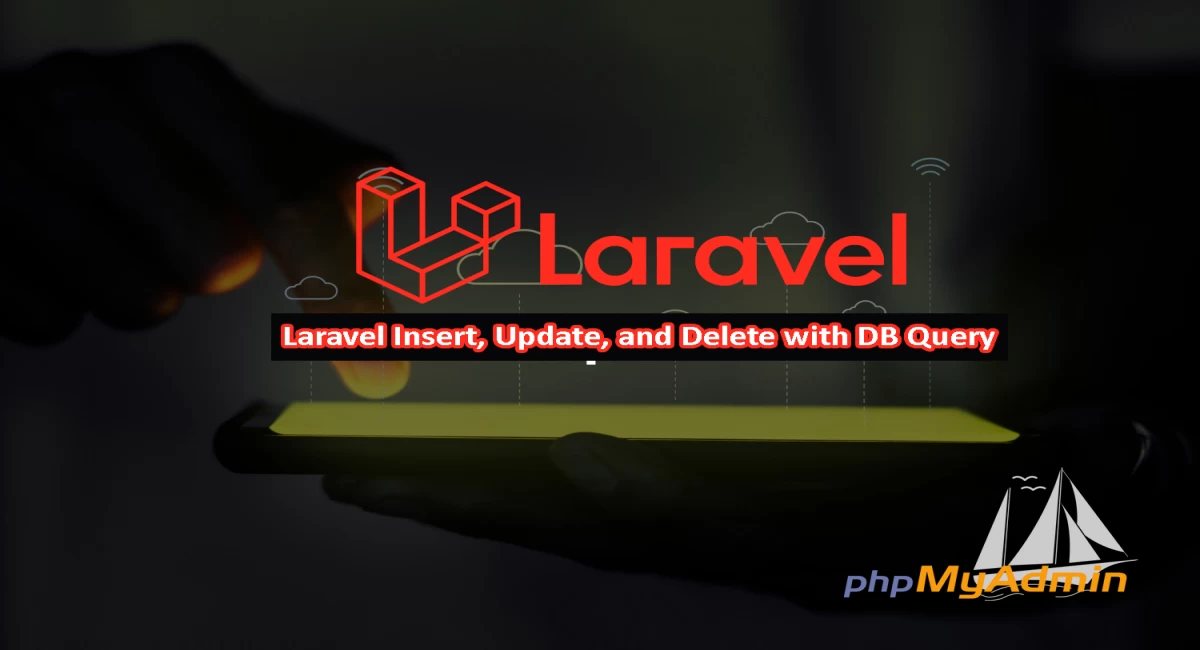
Laravel Insert, Update, and Delete with DB Query
In Laravel, you can use the DB Query builder to perform basic database operations like inserting, updating, and deleting data. This is useful when you don't need to use models or when you want to execute raw SQL queries.
Inserting Data
To insert data into a database table using the DB Query builder, you can use the insert()
method. Here's an example:
$data = ['name' => 'John Doe','email' => 'johndoe@example.com','password' => bcrypt('secret'),];
DB::table('users')->insert($data);
In this example, we're inserting a new user into the users
table. We first define an array of data that we want to insert, which includes the user's name, email, and password (which is hashed using Laravel's bcrypt()
function). We then use the insert()
method to insert the data into the table.
Updating Data
To update data in a database table using the DB Query builder, you can use the update()
method. Here's an example:
DB::table('users')
->where('id', 1)
->update(['name' => 'Jane Doe']);
In this example, we're updating the name of the user with an ID of 1. We use the where()
method to specify which row(s) to update, and then we use the update()
method to set the new name.
Deleting Data
To delete data from a database table using the DB Query builder, you can use the delete()
method. Here's an example:
DB::table('users')
->where('id', 1)
->delete();
In this example, we're deleting the user with an ID of 1. We use the where()
method to specify which row(s) to delete, and then we use the delete()
method to delete the data from the table.
Conclusion
The DB Query builder in Laravel provides a simple and convenient way to perform basic database operations without the need for models or raw SQL queries. By using the insert()
, update()
, and delete()
methods, you can easily insert, update, and delete data from a database table.
Comments
No comments yet.
Add Comment