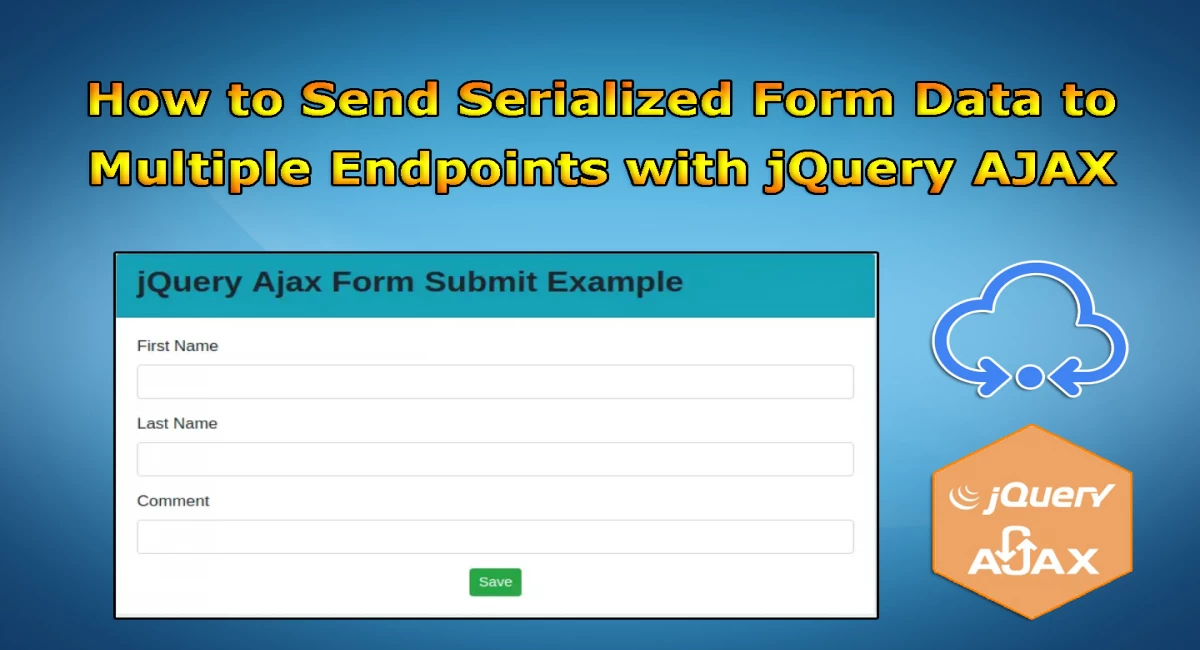
How to Send Serialized Form Data to Multiple Endpoints with jQuery AJAX
Submitting form data to a single endpoint is a common task in web development. But what if you need to send the same form data to multiple endpoints simultaneously? In this tutorial, we'll explore how to achieve this using jQuery AJAX and form data serialization.
Prerequisites
Before we begin, make sure you have the following:
- A basic understanding of HTML, JavaScript, and jQuery
- An HTML form with the necessary input fields
- The jQuery library included in your project
Step 1: Serialize the Form Data
To send form data to multiple endpoints, we first need to serialize the form data into a format that can be easily transmitted. jQuery provides a convenient method called serialize()
that converts the form data into a query string format.
var formData = $('form').serialize();
Step 2: Send the Serialized Data to Multiple Endpoints
Next, we'll use jQuery AJAX to send the serialized form data to multiple endpoints. Iterate through each endpoint URL and make a separate AJAX request for each.
$('form').submit(function(event) {
event.preventDefault();
var formData = $('form').serialize();
var endpoints = ['https://example.com/endpoint1', 'https://example.com/endpoint2', 'https://example.com/endpoint3'];
$.each(endpoints, function(index, endpoint) {
$.ajax({
url: endpoint,
type: 'POST',
data: formData,
success: function(response) {
console.log('Data submitted to ' + endpoint + ' successfully.');
},
error: function() {
console.error('Failed to submit data to ' + endpoint + '.');
}
});
});
});
Step 3: Handle the Responses
Finally, you can handle the responses from each endpoint within the AJAX success
or error
callback functions. You can log success messages or handle any errors that occur during the data submission.
Conclusion
In this tutorial, we've learned how to send serialized form data to multiple endpoints using jQuery AJAX. By serializing the form data and making separate AJAX requests for each endpoint, we can achieve simultaneous submission to multiple destinations. This technique can be useful in scenarios where you need to integrate with multiple APIs or services.
Remember to include the necessary error handling and validation mechanisms to ensure a robust and reliable form submission process.
Happy coding!
Comments
No comments yet.
Add Comment