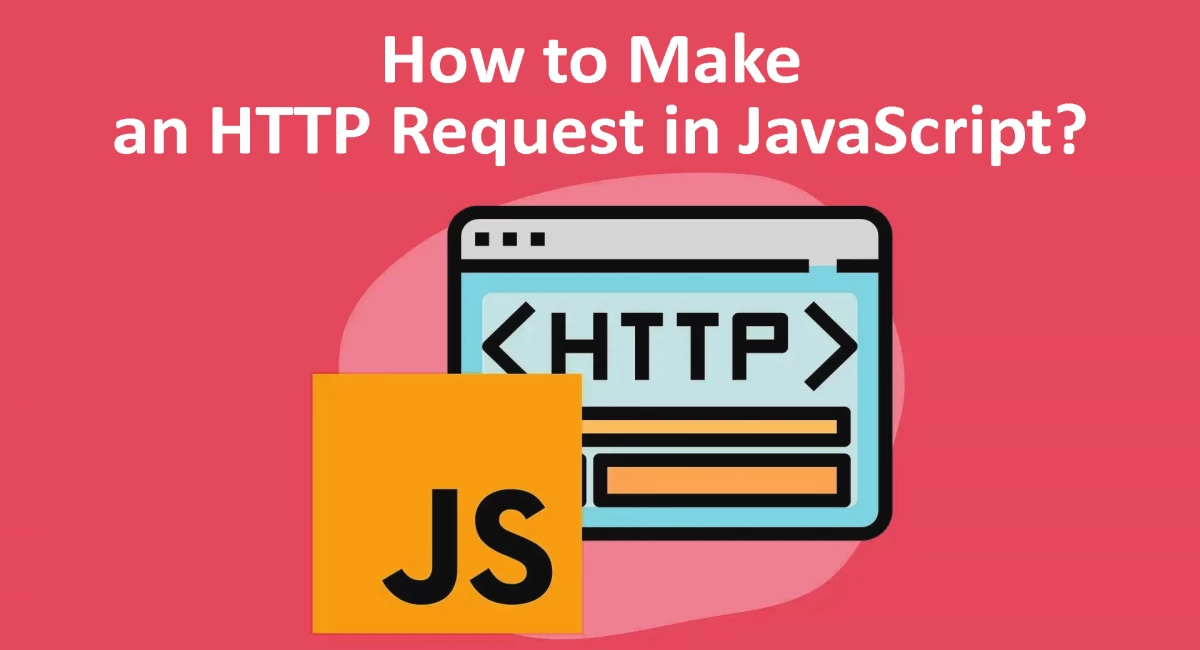
How to Make an HTTP Request in JavaScript
JavaScript is a versatile programming language that allows you to interact with web servers and retrieve data through HTTP requests. In this blog post, we will explore different methods for making HTTP requests in JavaScript and provide code examples for each approach.
1. Using the Fetch API
The Fetch API is a modern, promise-based approach for making HTTP requests in JavaScript. It provides a simple and concise syntax for performing various types of requests.
// Example HTTP GET request
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
// Process the response data
})
.catch(error => {
// Handle any errors
});
2. Utilizing XMLHttpRequest
The XMLHttpRequest object is another commonly used method for making HTTP requests in JavaScript. It provides more control and flexibility compared to the Fetch API, but with a slightly more verbose syntax.
// Example HTTP GET request
var xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.example.com/data', true);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
var data = JSON.parse(xhr.responseText);
// Process the response data
}
};
xhr.send();
3. Choosing the Appropriate HTTP Method
When making HTTP requests, it's important to select the appropriate HTTP method based on the desired action. The most commonly used methods are:
- GET: Retrieves data from the server
- POST: Submits data to the server
- PUT: Updates existing data on the server
- DELETE: Deletes data from the server
4. Handling Errors and Exceptions
When making HTTP requests in JavaScript, it's crucial to handle any potential errors and exceptions gracefully. This ensures that your application remains robust and provides a good user experience.
// Example error handling using Fetch API
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('HTTP error, status = ' + response.status);
}
return response.json();
})
.then(data => {
// Process the response data
})
.catch(error => {
// Handle any errors
});
Conclusion
Making HTTP requests in JavaScript is an essential skill for web developers. In this blog post, we covered two popular methods: the Fetch API and XMLHttpRequest. We also discussed the importance of choosing the appropriate HTTP method and handling errors effectively. By mastering these techniques, you'll be able to retrieve data from servers and build dynamic web applications.
Comments
No comments yet.
Add Comment