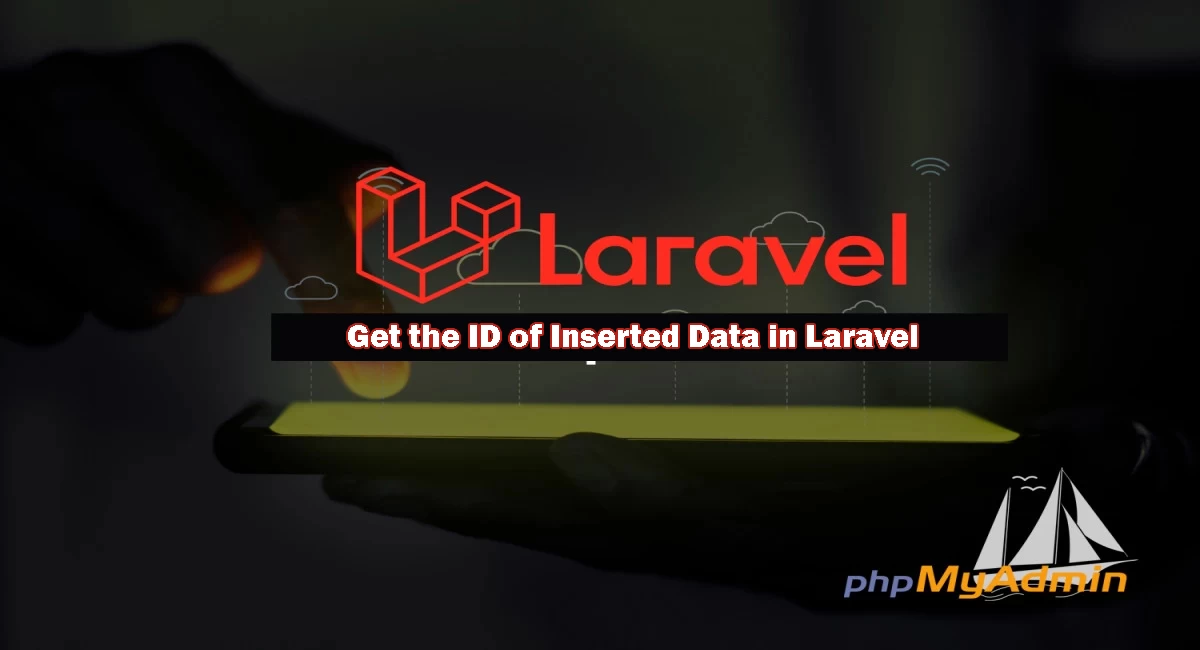
Get the ID of Inserted Data in Laravel | Tutorial
Are you using Laravel and wondering how to retrieve the ID of the data you just inserted into your database? Look no further! In this tutorial, we will guide you through the process of obtaining the ID of the inserted data using the power of Laravel's Eloquent model and DB facade. Let's get started!
Using the Eloquent Model to Retrieve the ID
Laravel's Eloquent model makes it incredibly simple to retrieve the ID of the inserted data. Follow the steps below:
$user = new User;
$user->name = 'John Doe';
$user->email = 'john@example.com';
$user->save();
$userId = $user->id;
In the above example, we create a new instance of the "User" model and set its attributes. By calling the "save" method, the data is inserted into the database. To access the ID of the newly inserted user, we can use the "$user->id" property. It's that easy!
Using the DB Facade for Easy ID Retrieval
Laravel also offers the DB facade for inserting data and retrieving the ID in a breeze. Follow these steps:
$data = [
'name' => 'Jane Smith',
'email' => 'jane@example.com',
];
$userId = DB::table('users')->insertGetId($data);
In the example above, we define an associative array containing the data we want to insert. By calling the "insertGetId" method on the "users" table using the DB facade, Laravel inserts the data and returns the ID of the newly inserted record. We simply store it in the "$userId" variable for further use. It's that simple and efficient!
Conclusion
Retrieving the ID of inserted data in Laravel is a crucial task, and with the help of the Eloquent model and DB facade, it becomes a seamless process. By following the steps outlined in this tutorial, you can easily obtain the ID of the inserted data and use it for related operations. Don't forget to handle any potential errors and exceptions to ensure smooth database operations.
Comments
No comments yet.
Add Comment