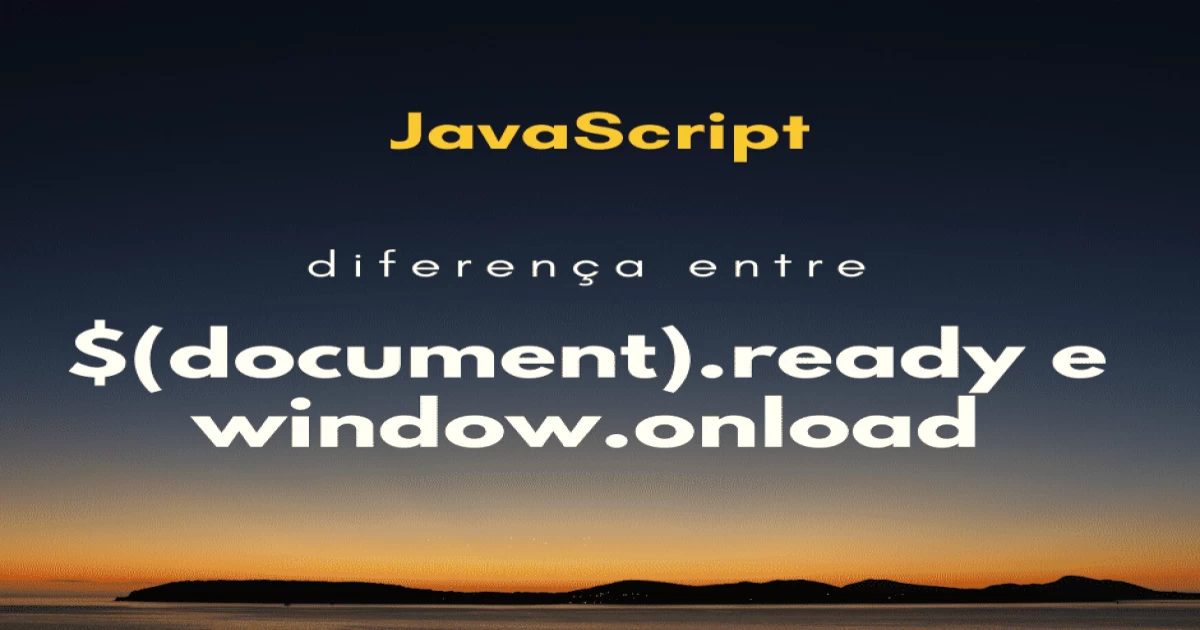
Understanding Document Ready jQuery, Window DOMContentLoaded JavaScript, and Window Onload JavaScript
When developing websites or web applications, it's crucial to ensure that all necessary resources, including HTML, CSS, and JavaScript, are loaded before displaying the page to users. Failing to do so can result in functionality issues and a poor user experience. To address this challenge, developers employ various techniques such as Document Ready in jQuery, DOMContentLoaded in JavaScript, and Window Onload in JavaScript.
Document Ready in jQuery: Ensuring Proper Resource Loading
jQuery is a widely used JavaScript library that simplifies HTML document traversal, event handling, and animation. Among its key features is the Document Ready function. This function is a jQuery event that triggers when the DOM tree, including CSS, JavaScript, and images, is fully loaded.
Here's how you can use it:
<script>
$(document).ready(function() {
// Code to execute after the DOM is ready
});
</script>
The code above creates a jQuery object that references the document and waits until it is fully loaded before executing the code within the function. This technique ensures that all HTML, CSS, and JavaScript elements are available for manipulation.
DOMContentLoaded in JavaScript: Handling Initial HTML Loading
The DOMContentLoaded event, a native JavaScript event, is similar to the Document Ready event in jQuery. It triggers when the initial HTML document has been completely loaded and parsed, without waiting for images, CSS, or subframes to finish loading.
Here's how you can use it:
<script>
document.addEventListener("DOMContentLoaded", function() {
// Code to execute after the DOM is ready
});
</script>
The above code adds an event listener to the document, waiting for the DOMContentLoaded event to trigger. Once triggered, the function inside the event listener is executed.
Window Onload in JavaScript: Handling Complete Resource Loading
The Window Onload event is a native JavaScript event that triggers when all resources on a web page, such as images, CSS, and JavaScript files, have finished loading.
Here's how you can use it:
<script>
window.onload = function() {
// Code to execute after the page is loaded
};
</script>
The code above assigns a function to the window.onload property, which is executed when all resources of the web page have finished loading.
Differences between Document Ready, DOMContentLoaded, and Window Onload:
The primary distinction between these events lies in their triggering times. The Document Ready and DOMContentLoaded events are triggered when the HTML document is loaded, while the Window Onload event is triggered when all resources, including images, CSS, and JavaScript files, have finished loading.
The Document Ready and DOMContentLoaded events are triggered earlier than the Window Onload event. They can be used to execute JavaScript code that manipulates the DOM elements before images or other resources are fully loaded. On the other hand, the Window Onload event should be used when the page's layout and appearance depend on the dimensions of images or other resources.
In summary, Document Ready in jQuery and DOMContentLoaded in JavaScript are employed to trigger events when the HTML document is loaded, while Window Onload is used when all resources, including images, CSS, and JavaScript files, are loaded. Understanding the distinctions between these events is crucial when developing web applications to ensure the website functions correctly and provides a positive user experience.
Comments
No comments yet.
Add Comment