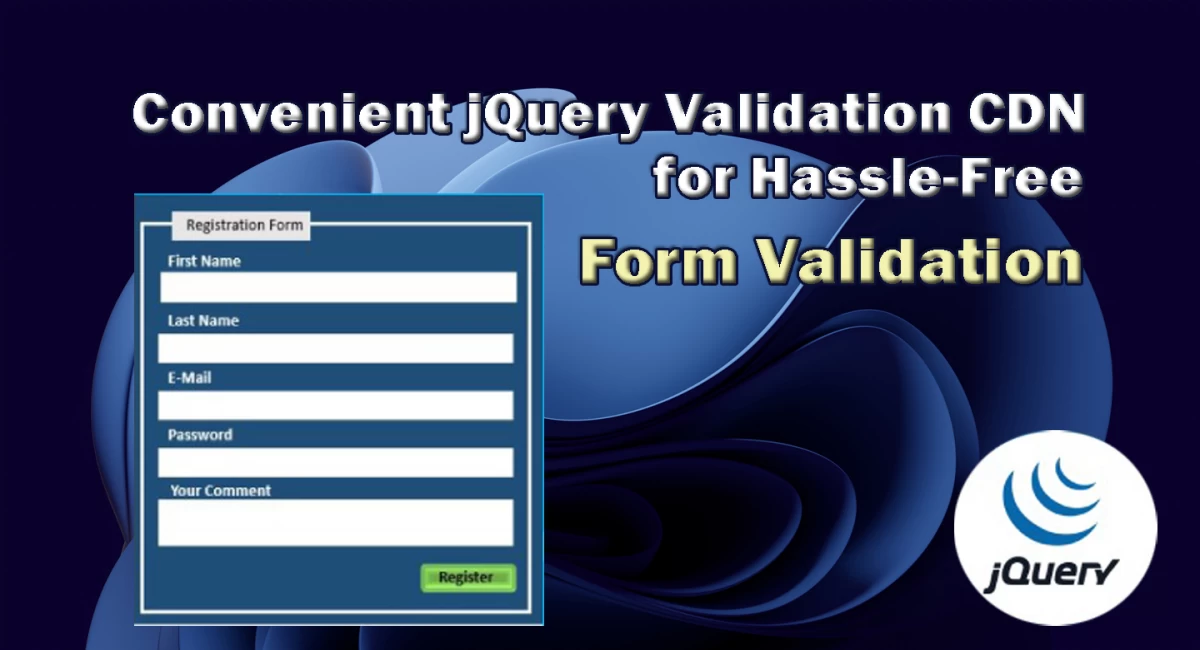
Convenient jQuery Validation CDN for Hassle-Free Form Validation
When it comes to validating user input in web forms, jQuery validation is a popular and convenient choice for developers. It provides a straightforward way to add form validation without writing complex JavaScript code from scratch. In this blog post, we'll explore how to implement hassle-free form validation using a convenient jQuery validation CDN.
What is jQuery validation?
jQuery validation is a JavaScript library that simplifies form validation. It offers a wide range of built-in validation rules and customizable error messages, making it easy to handle various types of user input. By including the jQuery validation CDN in your web project, you can leverage its powerful features without the need for local file hosting or manual installation.
Step-by-Step Guide to Using jQuery Validation CDN
Follow these steps to implement hassle-free form validation using the jQuery validation CDN:
- Include the jQuery library and the jQuery validation CDN in your HTML file:
- Add the necessary HTML markup for your form:
- Initialize the jQuery validation plugin and specify the validation rules:
- Add styling to indicate validation errors (optional):
- That's it! Now, when a user submits the form, jQuery validation will automatically validate the input based on the specified rules and display appropriate error messages if necessary.
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdn.jsdelivr.net/jquery.validation/1.19.3/jquery.validate.min.js"></script>
<form id="myForm">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<button type="submit">Submit</button>
</form>
$(document).ready(function() {
$('#myForm').validate({
rules: {
name: 'required',
email: {
required: true,
email: true
}
},
messages: {
name: 'Please enter your name',
email: {
required: 'Please enter your email address',
email: 'Please enter a valid email address'
}
}
});
});
label.error {
color: red;
}
Benefits of Using jQuery Validation CDN
Using a jQuery validation CDN offers several advantages:
- Convenience: The CDN eliminates the need for manual installation and hosting of the jQuery validation library.
- Performance: CDNs often have widespread server networks, resulting in faster loading times for the validation library.
- Reliability: CDNs are designed for high availability and can handle heavy traffic loads, ensuring consistent access to the validation library.
Conclusion
Implementing hassle-free form validation is made easy with the help of a convenient jQuery validation CDN. By following the steps outlined in this blog post, you can quickly add form validation to your web project without the need for complex JavaScript code. Enjoy the convenience, reliability, and performance benefits that come with using a jQuery validation CDN!
Comments
No comments yet.
Add Comment