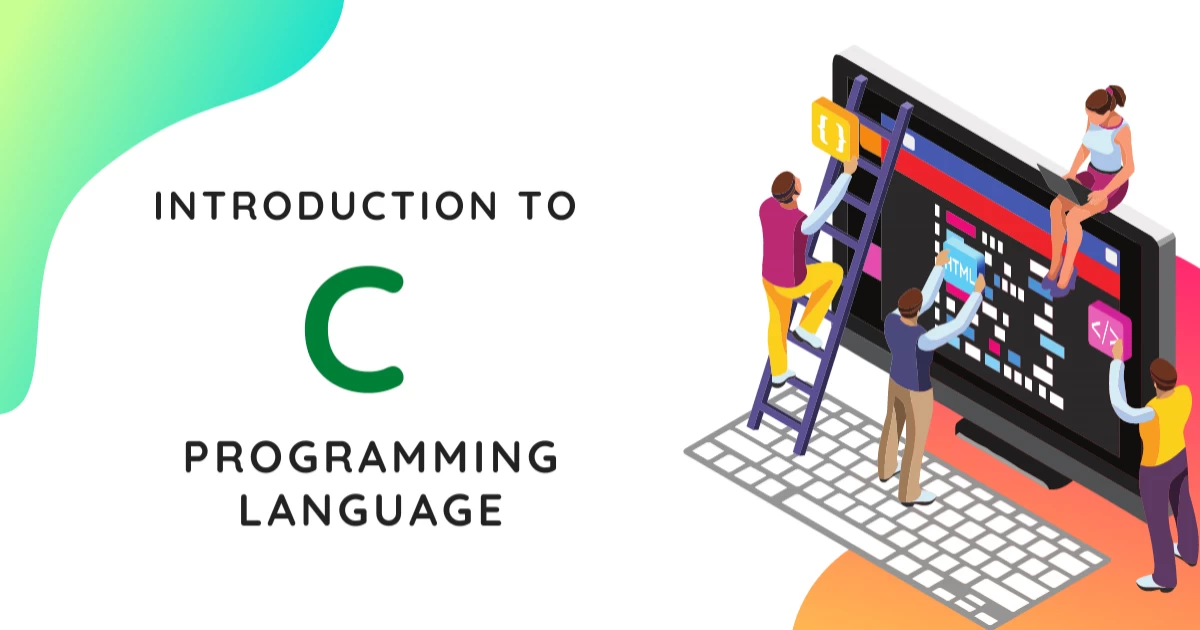
An Introduction to C Programming Language | Learn About C Programming Basics
C is a powerful programming language that is widely used for system programming, application development, and embedded systems. It was developed in the 1970s by Dennis Ritchie at Bell Labs and has since become one of the most popular programming languages in the world.
In this blog post, we will explore C programming language, providing a detailed explanation of what C is, how it works, and examples of its usage.
What is C Programming Language?
C is a general-purpose, high-level programming language that is used for developing operating systems, device drivers, and other low-level system programming tasks. It is also widely used for application development, including the development of desktop applications, web applications, and mobile apps.
C is a compiled language, which means that the source code is compiled into machine code that can be executed directly by the computer's CPU.
C is known for its efficiency, portability, and versatility. It is a low-level language that provides direct access to hardware resources, making it ideal for system programming tasks.
How does C Programming Language work?
C programming language works by compiling source code into machine code that can be executed directly by the computer's CPU. The source code is written in a text editor and saved in a file with a .c extension.
The C compiler takes the source code and generates an executable file that can be run on the computer. The compiler also performs syntax checking, error detection, and optimization of the code.
C programming language uses variables, functions, and control structures to create programs. Variables are used to store data, functions are used to perform operations on the data, and control structures are used to control the flow of the program.
Examples of C Programming Language
Here are a few examples of C programming language code:
Hello World
c#include <stdio.h>
int main() {
printf("Hello, World!");
return 0;
}
This is a simple program that prints "Hello, World!" to the console.
Variables
c#include <stdio.h>
int main() {
int x = 5;
float y = 3.14;
char z = 'A';
printf("x = %d\n", x);
printf("y = %f\n", y);
printf("z = %c\n", z);
return 0;
}
This program demonstrates the use of variables in C. It declares an integer variable, a float variable, and a character variable, and then prints their values to the console.
Control Structures
c#include <stdio.h>
int main() {
int x = 5;
if (x > 0) {
printf("x is positive");
} else if (x < 0) {
printf("x is negative");
} else {
printf("x is zero");
}
return 0;
}
This program demonstrates the use of control structures in C. It uses an if statement to determine whether a variable is positive, negative, or zero.
Functions
c#include <stdio.h>
int square(int x) {
return x * x;
}
int main() {
int x = 5;
int y = square(x);
printf("%d squared is %d", x, y);
return 0;
}
This program demonstrates the use of functions in C. It defines a function that squares a number and then calls the function to square a variable and print the result to the console.
Comments
No comments yet.
Add Comment