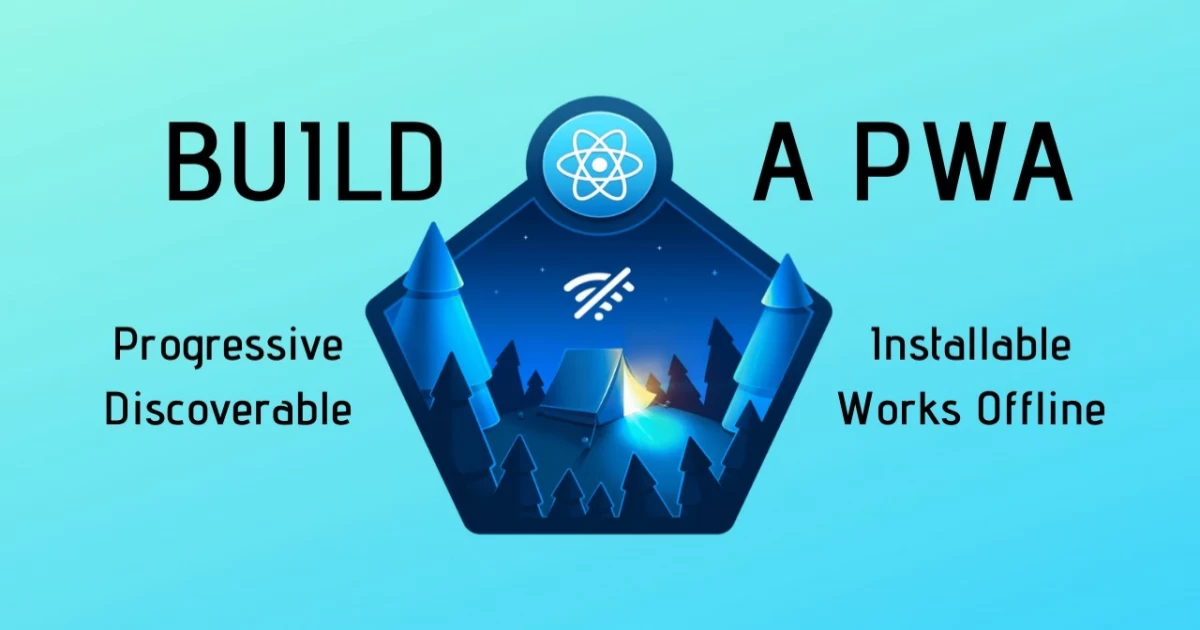
Building a Basic PWA: Offline Caching, Logo, and Installation Features
Progressive Web Apps, or PWAs, are web applications that combine the best of both worlds: the versatility of the web and the functionality of native apps. PWAs can be installed on a user's device, have access to device capabilities such as the camera and push notifications, and work offline. In this article, we'll explore how to build a basic PWA from scratch, including adding a logo, offline caching, and installing the app on a user's device.
How to make a basic PWA
To make a basic PWA, you need to add offline support to your app using a service worker. This can be done by caching your app's assets, such as HTML, CSS, JavaScript, and images, and serving them when the user is offline. You can define which files and resources to cache in your service worker, and how to handle network requests when offline. By providing offline support, you can create a reliable and fast experience for your users, and make your PWA feel more like a native app.
Here's the steps to build a basic PWA:
Step 1: Setting up the basic project structure
To get started, create a new folder and add an index.html file. Inside the index.html file, add the basic HTML5 structure. Then, create a new folder named "assets" and add a logo image file in it. We will use this logo image file as our PWA logo.
Step 2: Adding a manifest file
To create a PWA, we need to define a manifest file. The manifest file is a JSON file that describes the PWA's metadata, such as its name, icons, and startup URL. Create a new file named "manifest.json" in the root folder of your project and add the following JSON code:
{
"name": "My PWA App",
"short_name": "My App",
"icons": [
{
"src": "/assets/logo.png",
"sizes": "192x192",
"type": "image/png"
}
],
"start_url": "/index.html",
"display": "standalone",
"theme_color": "#ffffff",
"background_color": "#ffffff"
}
Step 3: Adding service worker
The service worker is the backbone of a PWA. It enables offline caching, push notifications, and background sync. To add a service worker, create a new file named "sw.js" in the root folder of your project and add the following code:
self.addEventListener('install', event => {
console.log('Service worker installed');
event.waitUntil(
caches.open('my-cache').then(cache => {
return cache.addAll([
'/',
'/index.html',
'/assets/logo.png',
'/sw.js'
]);
})
);
});
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request).then(response => {
return response || fetch(event.request);
})
);
});
Please ensure that you add the file "sw.js" to the root directory of your project as this is a commonly encountered error.
Step 4: Registering service worker
To register the service worker, add the following script tag at the bottom of your index.html file:
<script>
if ('serviceWorker' in navigator) {
window.addEventListener('load', () => {
navigator.serviceWorker.register('/sw.js')
.then(registration => {
console.log('Service worker registered');
})
.catch(error => {
console.error('Service worker registration failed:', error);
});
});
}
</script>
Step 5: Adding installation prompt
To allow users to install our PWA on their device, we need to add an installation prompt. Add the following script tag in your index.html file:
<script>
window.addEventListener('beforeinstallprompt', event => {
event.preventDefault();
const deferredPrompt = event;
const installButton = document.querySelector('#install-button');
installButton.addEventListener('click', event => {
deferredPrompt.prompt();
deferredPrompt.userChoice.then(choiceResult => {
console.log(choiceResult.outcome);
deferredPrompt = null;
});
});
installButton.hidden = false;
});
</script>
Then, add a button with the id "install-button" and hide it by default:
<button id="install-button" hidden>Install PWA</button>
Step 6: Testing the PWA
Finally, we need to test our PWA to make sure it works as expected. Open the project in your browser and try the following:
- Go offline and reload the page to make sure the cached resources are used.
- Add the app to your home screen by clicking the "Install PWA" button and make sure it launches in standalone mode.
Conclusion
Next, try going offline and refreshing the page. You should still see the app's content because the service worker has cached it. Try installing the PWA by clicking the "Add to Home Screen" button in the browser's menu. You should see your app's icon on your device's home screen.
Congratulations! You have successfully created a basic PWA with offline caching, logo, and installation features. From here, you can explore additional PWA features such as push notifications, background sync, and more advanced caching strategies.
Comments
No comments yet.
Add Comment