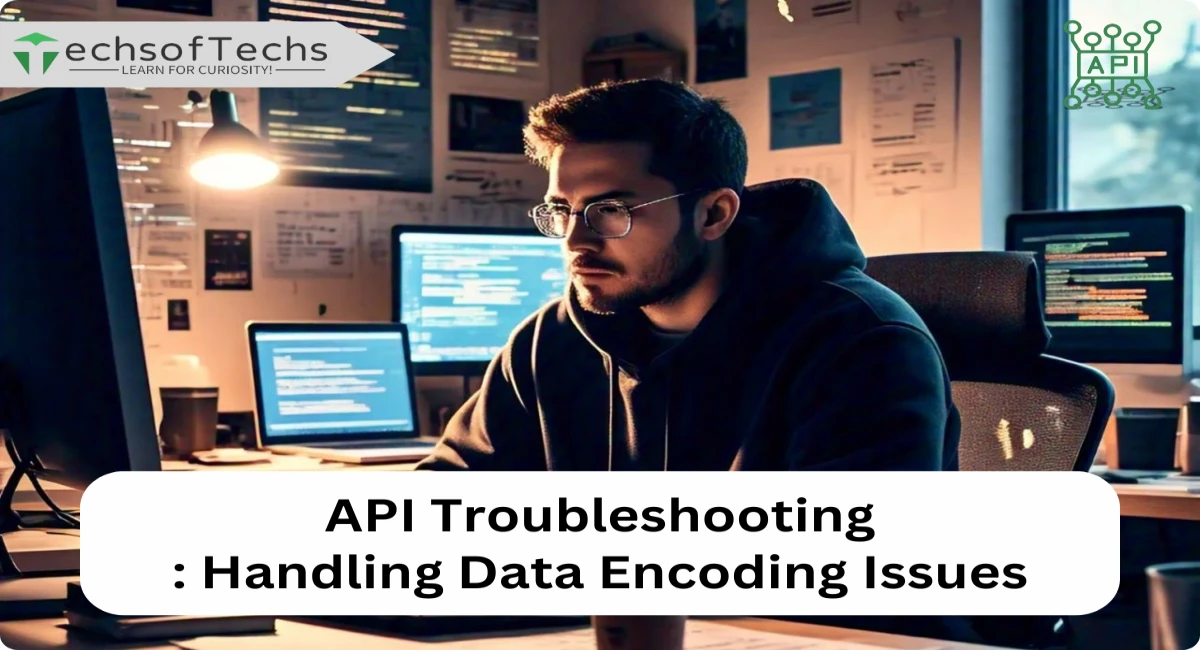
API Troubleshooting: Handling Data Encoding Issues
APIs are the unsung heroes of modern apps, quietly powering seamless communication between systems. But what happens when an API that’s been working fine suddenly starts returning empty responses? Let’s dive into one of the sneakiest culprits: data encoding issues.
Why Does Your API Return Empty Responses?
Ever tested your API, found everything perfect, and then hit production only to see []
—an empty JSON array? No errors, no crashes—just... nothing. Frustrating, right? The answer might lie in character encoding.
What’s Character Encoding & Why Does It Matter?
APIs work with text data, which needs to be encoded correctly (typically in UTF-8
) for functions like json_encode
to process it properly. If even one rogue character is non-UTF-8, json_encode
can silently fail, returning an empty response. Sneaky, huh?
How to Detect Encoding Problems?
Want to know if encoding is the problem? Use json_last_error()
. Here’s a quick check:
$json = json_encode($data);
if ($json === false) {
echo 'JSON Error: ' . json_last_error_msg();
}
Pro Tip: Always use error logging in production to catch encoding errors early!
How to Fix Encoding Issues in APIs?
Once you know encoding is the troublemaker, here’s how to fix it:
1. Validate Character Encoding
Check if your data is in UTF-8
and convert it if it isn’t:
foreach ($data as &$item) {
foreach ($item as &$value) {
if (!mb_detect_encoding($value, 'UTF-8', true)) {
$value = utf8_encode($value);
}
}
}
2. Sanitize Your Inputs
Always ensure database fields and user inputs are in UTF-8
. For MySQL users, set the collation to utf8mb4_general_ci
for consistent encoding.
3. Use Error Logging
Don’t skip logging! It’s your best friend when things go wrong:
$json = json_encode($data);
if ($json === false) {
error_log('JSON Error: ' . json_last_error_msg());
}
UTF-8
?
Tips to Prevent Future API Woes
Don’t wait for encoding issues to strike again! Follow these best practices:
- Always default to
UTF-8
for your app and database. - Validate and sanitize all inputs as early as possible.
- Test your API with diverse, real-world datasets to catch edge cases.
Ready to Debug Smarter?
Encoding issues might seem like a small detail, but they can cause big headaches. With proper validation, logging, and prevention strategies, you can make your APIs bulletproof.
Bonus: Want to dive deeper into API debugging? Check out our guide on handling JSON
errors effectively.
Comments
No comments yet.
Add Comment