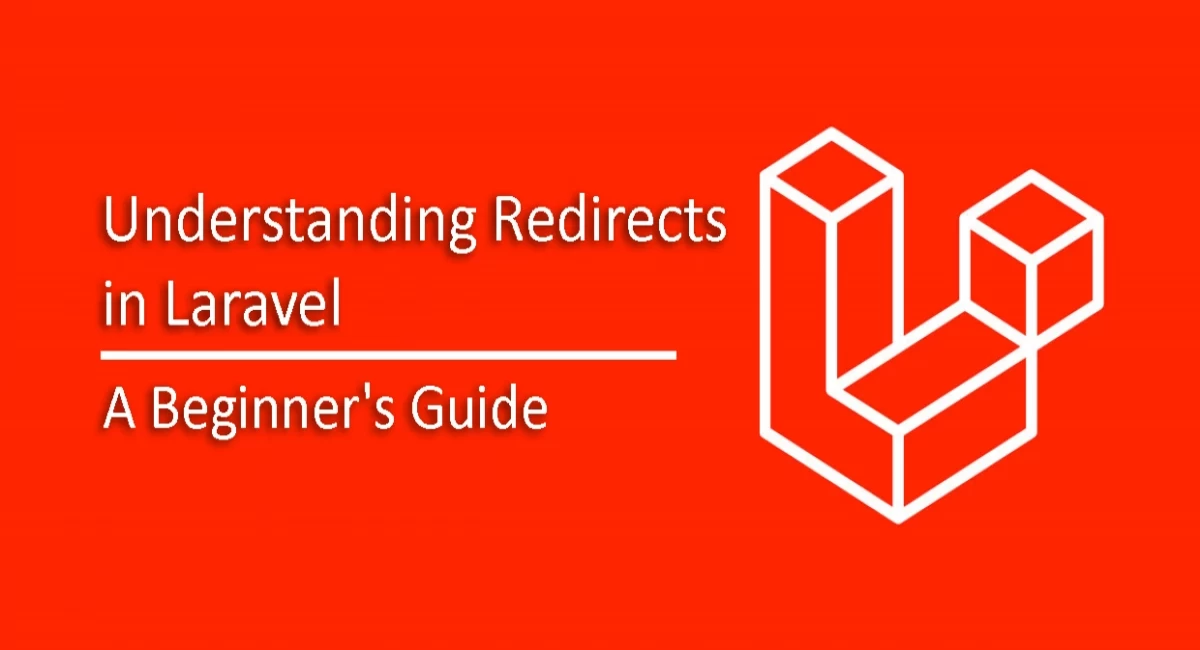
Understanding Redirects in Laravel
👋 Hi there! In this blog, we're going to talk about redirects in Laravel and how they can be used to direct users to the correct page or action.
🔀 Redirecting is a process of sending a user from one page to another. Laravel provides several ways to redirect users, such as the redirect() function and the Redirect class.
👉 Let's start with the redirect() function, which is used to create a new redirect response. The redirect() function takes a URL as an argument and returns a RedirectResponse object. Here's an example:
return redirect('dashboard');
This code will redirect the user to the dashboard page.
👉 The Redirect class is another way to create a redirect response. This class provides several methods to create different types of redirects. Here are some examples:
// Redirect to a named route
return redirect()->route('home');
// Redirect to a URL with parameters
return redirect()->to('profile/' . $user->id);
// Redirect with a status code
return redirect('dashboard')->with('status', 'Profile updated!');
In the first example, we're redirecting to a named route home. The second example shows how to redirect to a URL with parameters. The third example shows how to redirect with a status code and flash data.
👉 Laravel also provides some shortcuts for common redirects. Here are some examples:
// Redirect back to the previous URL
return back();
// Redirect to the previous URL with input
return back()->withInput();
// Redirect to a 404 error page
abort(404);
The back() function redirects the user to the previous URL, while back()->withInput() redirects with the old input data. The abort(404) function returns a 404 error page.
👉 Another useful feature of Laravel's redirects is the ability to redirect with flash data. Flash data is a type of session data that only lasts for one request. Here's an example:
return redirect('dashboard')->with('status', 'Profile updated!');
In this example, we're redirecting to the dashboard page with a status message. This message will be available in the session for the next request.
👉 Finally, it's worth noting that you can also redirect with named routes and controller actions. Here are some examples:
// Redirect to a named route with parameters
return redirect()->route('profile', ['id' => $user->id]);
// Redirect to a controller action
return redirect()->action([ProfileController::class, 'index']);
In the first example, we're redirecting to a named route profile with the parameter id. The second example shows how to redirect to a controller action.
That's it for this blog! We hope you found it helpful in understanding redirects in Laravel. Remember, redirects are an essential part of any web application, so make sure you're familiar with how they work!
Comments
No comments yet.
Add Comment