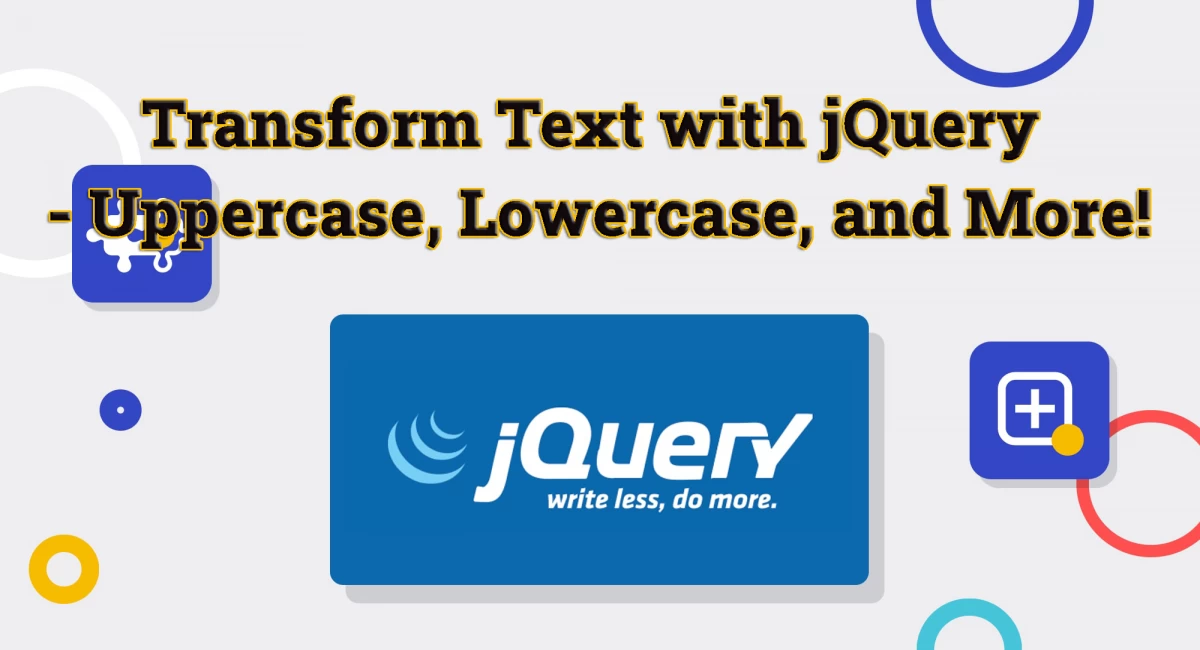
Learn to Transform Text with jQuery - Uppercase, Lowercase, and More!
Transforming text is a common requirement in web development. Whether you need to capitalize the first letter of each word, make all text uppercase or lowercase, or perform other transformations, jQuery can help you achieve this quickly and easily.
Changing Case with jQuery
There are two ways to change the case of text using jQuery. The first method involves using CSS to apply text transformations:
/* CSS */
.uppercase {
text-transform: uppercase;
}
.lowercase {
text-transform: lowercase;
}
.capitalize {
text-transform: capitalize;
}
To use these CSS classes with jQuery, you can simply add or remove them from the element you want to transform:
// jQuery
$('.my-element').addClass('uppercase');
$('.my-element').removeClass('lowercase');
The second method involves using jQuery functions to change the case of text. For example, to convert all text in an element to uppercase, you can use the .toUpperCase()
function:
// jQuery
var text = $('.my-element').text();
$('.my-element').text(text.toUpperCase());
To convert all text to lowercase, you can use the .toLowerCase()
function:
// jQuery
var text = $('.my-element').text();
$('.my-element').text(text.toLowerCase());
To capitalize the first letter of each word, you can use a combination of jQuery functions:
// jQuery
var text = $('.my-element').text();
var capitalized = text.toLowerCase().replace(/(^|\s)\S/g, function (firstLetter) {
return firstLetter.toUpperCase();
});
$('.my-element').text(capitalized);
Other Text Transformations with jQuery
In addition to changing case, there are many other ways to transform text using jQuery. For example, you can remove all whitespace from a string using the .trim()
function:
// jQuery
var text = $('.my-element').text();
var trimmed = text.trim();
$('.my-element').text(trimmed);
You can also replace specific characters or substrings using the .replace()
function:
// jQuery
var text = $('.my-element').text();
var replaced = text.replace('foo', 'bar');
$('.my-element').text(replaced);
Finally, you can use regular expressions to perform more complex text transformations. For example, you can remove all non-alphanumeric characters from a string using the following code:
// jQuery
var text = $('.my-element').text();
var cleaned = text.replace(/\W/g, '');
$('.my-element').text(cleaned);
Conclusion
With jQuery, transforming text is a breeze. Whether you need to change case, remove whitespace, or perform other transformations, jQuery provides a wide range of functions and techniques to help you achieve your goals. By incorporating these techniques into your web development workflow, you can create cleaner, more consistent, and more professional-looking websites and applications.
Comments
No comments yet.
Add Comment