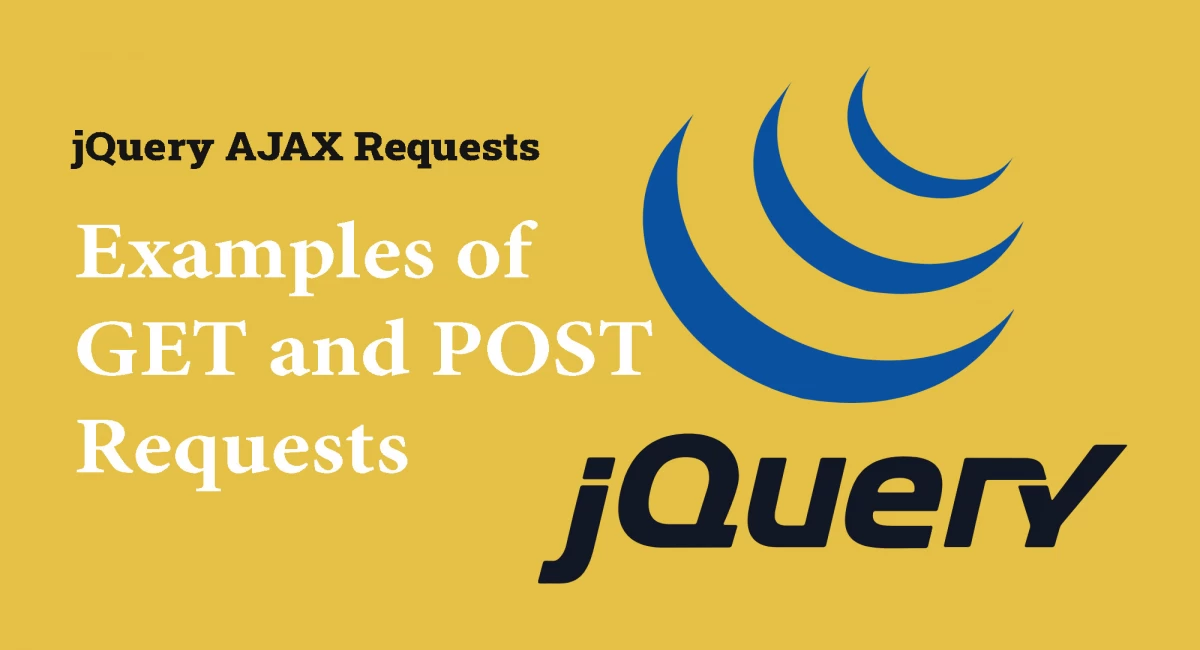
jQuery AJAX Requests - Examples of GET and POST Requests
jQuery is a popular JavaScript library that makes it easy to perform common web development tasks, such as making AJAX requests. AJAX stands for Asynchronous JavaScript and XML, and it allows web pages to send and receive data from a server without having to reload the entire page. In this blog, we will explore how to use jQuery to make GET and POST requests using AJAX.
Making a GET request with jQuery AJAX
To make a GET request using jQuery AJAX, you can use the $.ajax()
method. This method takes an object as an argument, which can include various options such as the URL to request and the type of request to make. Here is an example of how to make a GET request using jQuery AJAX:
$.ajax({
url: "https://api.example.com/data",
type: "GET",
success: function(data) {
// Do something with the data
},
error: function() {
// Handle errors
}
});
In this example, we are making a GET request to the URL "https://api.example.com/data"
. If the request is successful, the success
function will be called with the data that was returned by the server. If there is an error, the error
function will be called.
Making a POST request with jQuery AJAX
To make a POST request using jQuery AJAX, you can again use the $.ajax()
method, but with a different type
option. Additionally, you will need to include data to send with the request. Here is an example of how to make a POST request using jQuery AJAX:
$.ajax({
url: "https://api.example.com/data",
type: "POST",
data: {
name: "John",
email: "[email protected]"
},
success: function(data) {
// Do something with the data
},
error: function() {
// Handle errors
}
});
In this example, we are making a POST request to the URL "https://api.example.com/data"
. We are also including data to send with the request, in this case, the name and email of a person. If the request is successful, the success
function will be called with the data that was returned by the server. If there is an error, the error
function will be called.
Comments
No comments yet.
Add Comment