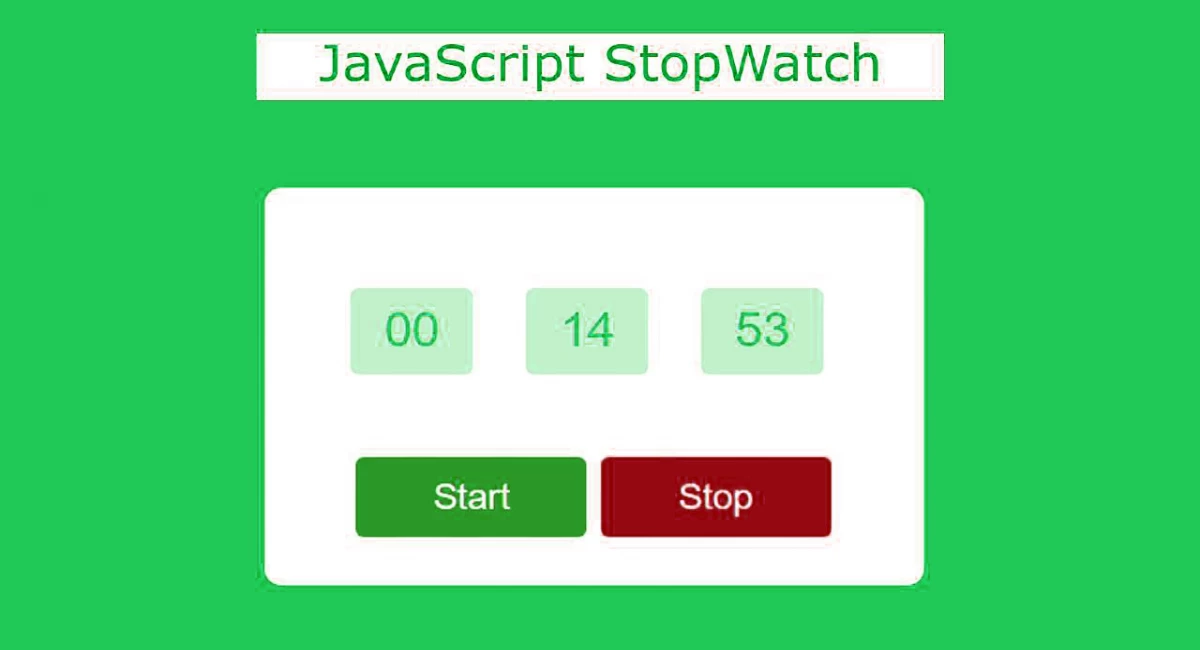
How to Make an Increasing Timer with Start-Stop Function in jQuery
Timers are an important part of web development, and jQuery provides an easy and efficient way to implement them. In this blog post, we will learn how to create an increasing timer with a start-stop function using jQuery.
First, we need to create a simple HTML page with a button to start and stop the timer and a span to display the time. Here is the HTML code:
<!DOCTYPE html>
<html>
<head>
<title>jQuery Timer</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="timer.js"></script>
</head>
<body>
<button id="start">Start</button>
<button id="stop">Stop</button>
<span id="time">00:00:00</span>
</body>
</html>
In this code, we have included the jQuery library and a JavaScript file called timer.js
, which we will create later.
Now let's move to the timer.js
file and start implementing the timer logic. First, we need to define a variable to keep track of the time:
var seconds = 0;
var minutes = 0;
var hours = 0;
Next, we need to define a function to update the time display. This function will take the current values of seconds, minutes, and hours and format them into a string in the format "hh:mm:ss". Here's the code for this function:
function updateTime() {
var timeString = "";
if (hours < 10) timeString += "0";
timeString += hours + ":";
if (minutes < 10) timeString += "0";
timeString += minutes + ":";
if (seconds < 10) timeString += "0";
timeString += seconds;
$("#time").text(timeString);
}
Now we need to define two functions: one to start the timer and another to stop it. The start function will use the setInterval()
function to call a function that increments the time values every second. Here's the code for the start function:
var interval;
function startTimer() {
interval = setInterval(function() {
seconds++;
if (seconds == 60) {
seconds = 0;
minutes++;
if (minutes == 60) {
minutes = 0;
hours++;
}
}
updateTime();
}, 1000);
}
The setInterval()
function takes two arguments: a function to be executed every x milliseconds, and the number of milliseconds to wait between each execution. In this case, we are passing an anonymous function that increments the time values and calls the updateTime()
function every second.
The stop function simply calls the clearInterval()
function to stop the timer:
function stopTimer() {
clearInterval(interval);
}
Finally, we use jQuery to add click event handlers to the start and stop buttons:
$(document).ready(function() {
$("#start").click(function() {
startTimer();
});
$("#stop").click(function() {
stopTimer();
});
});
And that's it! We have created an increasing timer with a start-stop function using jQuery. You can customize the code further to add features such as a reset button or to style the timer display to fit your website's design. Happy coding!
Comments
No comments yet.
Add Comment