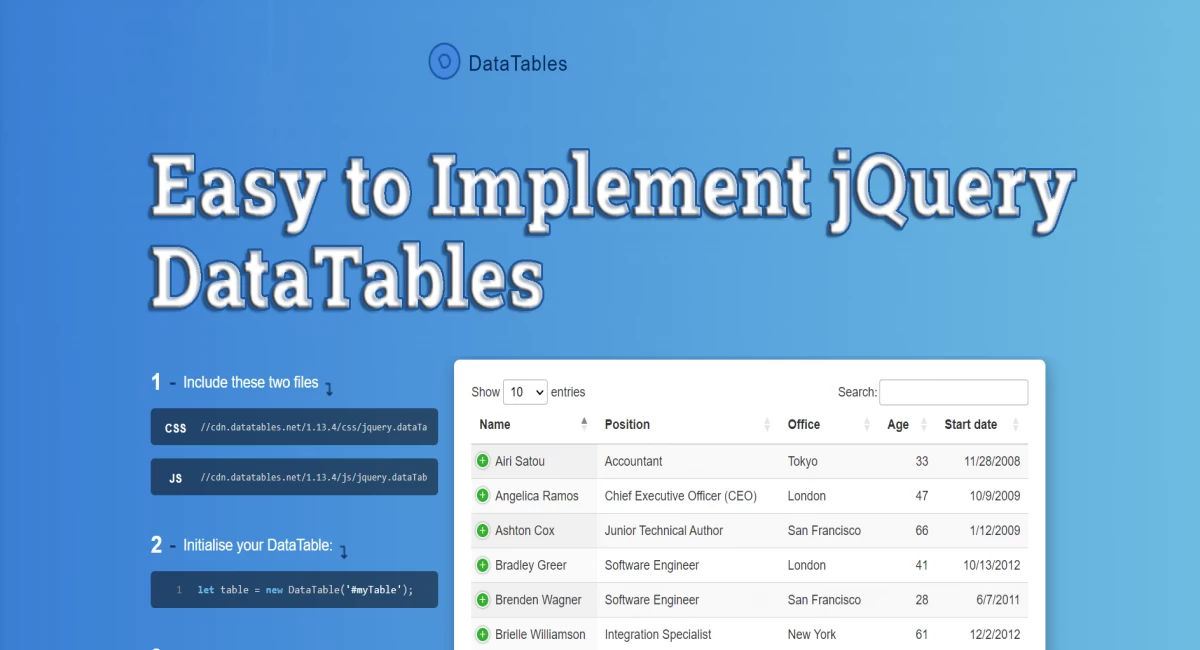
Easy to Implement jQuery DataTables
jQuery DataTables is a popular plugin that can turn any HTML table into an interactive and customizable grid. It provides features such as sorting, filtering, pagination, and searching out of the box, making it easy to work with large amounts of data.
Getting Started
The first step is to include the necessary files in your HTML document. You will need to add the following scripts to the <head>
section:
<link rel="stylesheet" href="https://cdn.datatables.net/1.10.24/css/jquery.dataTables.min.css">
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script>
<script src="https://cdn.datatables.net/1.10.24/js/jquery.dataTables.min.js"></script>
Once you have included the necessary files, make sure your html table structure is like this:
<table id="myTable">
<thead>
<tr>
<th>Name</th>
<th>Email</th>
<th>Phone</th>
</tr>
</thead>
<tbody>
<tr>
<td>John Doe</td>
<td>johndoe@example.com</td>
<td>1234567890</td>
</tr>
<tr>
<td>Jane Doe</td>
<td>janedoe@example.com</td>
<td>0987654321</td>
</tr>
</tbody>
</table>
You can initialize the DataTable by calling the .DataTable()
function on the table element. For example, if you have a table with the ID "myTable", you would call the function like this:
$(document).ready(function() {
$('#myTable').DataTable();
});
That's it! You now have a fully functional DataTable that you can customize to fit your needs.
Customization
One of the benefits of using DataTables is its flexibility. You can customize almost every aspect of the grid to match your design and functionality requirements. Here are a few examples:
Sorting
By default, DataTables will enable sorting on all columns. However, you can disable sorting on a specific column by adding the data-orderable="false"
attribute to the corresponding <th>
element. For example:
<thead>
<tr>
<th>Name</th>
<th data-orderable="false">Actions</th>
</tr>
</thead>
Pagination
By default, DataTables will display 10 rows per page. You can customize this value by using the iDisplayLength
option. For example, to display 25 rows per page, you would initialize the DataTable like this:
$(document).ready(function() {
$('#myTable').DataTable({
"iDisplayLength": 25
});
});
Searching
By default, DataTables will enable searching on all columns. However, you can disable searching on a specific column by adding the data-searchable="false"
attribute to the corresponding <th>
element. For example:
<thead>
<tr>
<th>Name</th>
<th data-searchable="false">Actions</th>
</tr>
</thead>
Conclusion
jQuery DataTables is a powerful and easy-to-use plugin that can enhance the functionality of your HTML tables. With just a few lines of code, you can add sorting, filtering, pagination , and searching to your tables, and customize them to fit your design and functionality requirements. Additionally, DataTables has extensive documentation and an active community that can help you with any questions or issues you may encounter.
So, if you're looking to add some interactivity and customization to your tables, give jQuery DataTables a try!
Comments
No comments yet.
Add Comment